Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
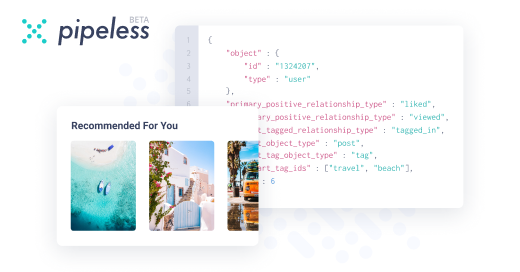
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "lambda"
-
I'm a self-taught 19-year-old programmer. Coding since 10, dropped out of high-school and got fist job at 15.
In the the early days I was extremely passionate, learning SICP, Algorithms, doing Haskell, C/C++, Rust, Assembly, writing toy compilers/interpreters, tweaking Gentoo/Arch. Even got a lambda tattoo on my arm after learning lambda-calculus and church numerals.
My first job - a company which raised $100,000 on kickstarter. The CEO was a dumb millionaire hippie, who was bored with his money, so he wanted to run a company even though he had no idea what he was doing. He used to talk about how he build our product, even tho he had 0 technical knowledge whatsoever. He was on news a few times which was pretty cringeworthy. The company had only 1 programmer (other than me) who was pretty decent.
We shipped the project, but soon we burned through kickstart money and the sales dried off. Instead of trying to aquire customers (or abandoning the project), boss kept looking for investors, which kept us afloat for an extra year.
Eventually the money dried up, and instead of closing gates, boss decreased our paychecks without our knowledge. He also converted us from full-time employees to "contractors" (also without our knowledge) so he wouldn't have to pay taxes for us. My paycheck decreased by 40% by I still stayed.
One day, I was trying to burn a USB drive, and I did "dd of=/dev/sda" instead of sdb, therefore wiping out our development server. They asked me to stay at company, but I turned in my resignation letter the next day (my highest ever post on reddit was in /r/TIFU).
Next, I found a job at a "finance" company. $50k/year as a 18-year-old. CEO was a good-looking smooth-talker who made few million bucks talking old people into giving him their retirement money.
He claimed he changed his ways, and was now trying to help average folks save money. So far I've been here 8 month and I do not see that happening. He forces me to do sketchy shit, that clearly doesn't have clients best interests in mind.
I am the only developer, and I quickly became a back-end and front-end ninja.
I switched the company infrastructure from shitty drag+drop website builder, WordPress and shitty Excel macros into a beautiful custom-written python back-end.
Little did I know, this company doesn't need a real programmer. I don't have clear requirements, I get unrealistic deadlines, and boss is too busy to even communicate what he wants from me.
Eventually I sold my soul. I switched parts of it to WordPress, because I was not given enough time to write custom code properly.
For latest project, I switched from using custom React/Material/Sass to using drag+drop TypeForms for surveys.
I used to be an extremist FLOSS Richard Stallman fanboy, but eventually I traded my morals, dreams and ideals for a paycheck. Hey, $50k is not bad, so maybe I shouldn't be complaining? :(
I got addicted to pot for 2 years. Recently I've gotten arrested, and it is honestly one of the best things that ever happened to me. Before I got arrested, I did some freelancing for a mugshot website. In un-related news, my mugshot dissapeared.
I have been sober for 2 month now, and my brain is finally coming back.
I know average developer hits a wall at around $80k, and then you have to either move into management or have your own business.
After getting sober, I realized that money isn't going to make me happy, and I don't want to manage people. I'm an old-school neck-beard hacker. My true passion is mathematics and physics. I don't want to glue bullshit libraries together.
I want to write real code, trace kernel bugs, optimize compilers. Albeit, I was boring in the wrong generation.
I've started studying real analysis, brushing up differential equations, and now trying to tackle machine learning and Neural Networks, and understanding the juicy math behind gradient descent.
I don't know what my plan is for the future, but I'll figure it out as long as I have my brain. Maybe I will continue making shitty forms and collect paycheck, while studying mathematics. Maybe I will figure out something else.
But I can't just let my brain rot while chasing money and impressing dumb bosses. If I wait until I get rich to do things I love, my brain will be too far gone at that point. I can't just sell myself out. I'm coming back to my roots.
I still feel like after experiencing industry and pot, I'm a shittier developer than I was at age 15. But my passion is slowly coming back.
Any suggestions from wise ol' neckbeards on how to proceed?32 -
Worst experience with cs profs? Oh boy....
Databases lab: "You'll need to work of this snippet, if your IDE tells you it's deprecated you don't need to care about it"
If you want to imagine the quality of the code base we were expected to work upon just think about that attached xkcd comic, basically an undecipherable black box.
The instructions where at the same time micro managing everything (he gave us frickin variable names to use, and no good ones, no the database connection had to be called datbc, yeah very descriptive) and yet so obfuscated that I'm not completely sure he didn't resurrect Kant himself to ghostwrite for him.
He also didn't like us to use any Java feature that was to 'modern', for example for each loops since "they offer no benefit over normal for loops".
Further, everything we wrote had to be documented with a relationship diagram and a uml. So far no problem if he hadn't invented his own flavor of both (which can be read about in his book).
Oh, and he almost failed me because I used a lambda expression in his 'code on paper' exam and this "arrows are a C command" I "must have been confused"... which is glorious coming from the guy who can't get operators and commands straight.1 -
Aren't lambda expressions just the most frustrating thing sometimes? But how can you not love them when they get the job done in one-liners? 😍2
-
A Developer is desperate: his java application servers are unresponsive, thousand of dead zombie threads are sucking all cpus, memory is leaking everywhere, garbage collector has gone crazy, the cluster sessions are fucked....
The Developer goes to the closest bridge, ties a stone to his neck and gets ready to jump.
Suddenly a bearded old man with a fiery look runs toward him, yelling:
- stop stop!!!! Your application is not scaling and misconfigured, your servers are melting, cpu usage is not sustainable anymore, but don't despair
The Developer, puzzled, looks at him:
-I've never seen you...how do you know...
- Hey, man, I'm the Devil. I know everything. All your problems are solved. I'll give you magic functions. They are called Lambda.
You'll never have to worry about your servers, scalability, security, configuration and shit.
The Developer seems astonished but relieved:
- Ok, sounds great! let's try it - suddenly suspicion creeps in - hmmmm but you are the Devil....so...you want something back, don't you?
(the Devil nods lightly with a diabolic smile)
- ...and...you want my soul, I guess...
- your soul??? come on!!! - the Devil burst in a laugh - we are in 2019. I don't care about your soul. I want your ass.
- What!???!!!?
- yes, I want to fuck your ass
The Developer, evaluates quickly the situation.
Few moments of pain or slight discomfort (?) in exchange for magic lambda. It could be worth. He accepts.
After a while of rough anal fucking, the devil asks
- Hey, how old are you anyway?
- 45, why?
- Oh jeeez...45!!!??? and you still believe in the devil?5 -
I work for a "Visionary" in our org. Motherfucker will use something for five minutes and decided it's the new-hot, and off we go chasing it.
"I did a Python + AWS tutorial last night, I get it. We need to start migrating everything to Lambda today."
A week later he hits his first error message that needs more than five minutes of work to solve and we're moving to a local Haskell stack.4 -
First time my laptop acted as a CV.
I've been in a personal project with my pal for like a three months. We meet sometimes at a cafe which is a very nice workplace, we often see more people with laptops, so we are not the only ones that thinks so.
My pal was waiting for me, he got a table early and then I arrived. there was a guy nearby us.
Me: (this guy has a newest new macbook pro, fucking riche)
-- I sit, put my laptop and start to work with my pal --
The guy starts looking at my stickers without hiding his doing at all. I noticed that instantly
Me: (Crap, he's gonna ask something :( )
-- I kept discussing stuff with my pal for like 5 minutes and then it happened. the guy stands up and... --
Guy: hey! how are you? sorry for bother, are you perhaps developers? I'm asking because I saw your stickers
Me: mmm yes
Guy: Do you have a job currently?
Me: We are in a project (No need to mention this is personal project and I got my full time job)
Guy: Oh, ok, no problem, you see I got a company, and currently we are looking for people to work with us, we want frontend developers with javascript skills preferable, but anything is welcome. Interviews starts next week, so if you are interested or know someone that could be, I'll give you my card and please write me at my mail if anything.
Me: got it, no problem.
-- I tried my best to hide my displeasure face(but I think I showed it a little), for him to being a riche with a new macbook pro, and you know, the interruption, I wanted to be focused while working in da project --
-- I got the card, I read it a bit, didn't dig into too much, there was stuff to do at the moment. the guy already returned to his chair and my friend --
Pal: Excuse me Mr Guy, what's the job tittle?
Me: (FUCK! dude!, we're working in our shit, don't give him more reason to try to scout us. we are behind the schedule and I need to explain this shit to you FFS)
Guy: Oh yes, will be frontend developer(again), but if you are a full stack that will be a plus too, we got some stuff with angular 1.x(ugh), and sencha touch(ugh) and ...(don't remember what else was it)
Pal: Ok and the job is full time in site? or are you open to work remotely
Me: (ok man, you sound interested, that makes me look interested too >:( )
Guy: preferable in site, but we would consider remotely depending on the person.
Pal: Good! thank you very much Mr. X
Guy: cool
-- Later on, like two hours, my friend goes to the counter for more coffee --
-- I text him: dude, I feel the guy will kidnap me or something --
-- then the guy start looking again at my laptop and... ---
Guy: hey! Jhon was your name right? Do you have experience with devops? I see your aws stickers
Me: yes
Guy: do you have experience with microservices?
Me: yes, a bit with lambda, also I've done some stuff with kubernetes, opsworks, rds and whatnot. no biggie
Guy: oh cool! we have a devops job too, there is a migration we need to do for an app to micro services. again if you are interested or know someone that it does. please mail me :)
Me: gotcha
There were no further interactions with Mr. Guy the rest of the day.
I'll be thrilled if someone ask me about my bee and puppycat sticker12 -
Supervisor: so you're going to write a perl script that will compile a jar that will be used to invoke a web service
Me: okay. What does the web service do?...
Supervisor: I'm not sure how it works. It'll just return a success or error code
Me: so I'm just going to invoke a black box?
Supervisor: that's a good way to think of it
Me: so how does the qa process work with this black box/how can we debug?
Supervisor: we don't have qa for it and we can't debug
What the fuck?!?!? You expect me to call a literal fucking black fucking box?!?! This isn't lambda calc you jabroni.2 -
Holy fucking dickballs, AWS cloud platform is one of the most UX unfriendly piles of fuckery I have witnessed.
It starts off okay and then you have to use it for many hours a day.
API gateway is assfucked backwards in its layout and how it displays. Why have things go horizontally across the screen rather than flow down so I can scroll. Also when I add a method to a resource why the god damn fuck do I need to select it from the smallest drop down imaginable when you have HALF MY POXY SCREEN TAKEN UP IN LITERAL WHITESPACE NEXT TO IT.
Now I get on to the dynamodb interface whoever designed must have been some form of insane cause it is as clunky as a donkey in clogs.
Finally, Lambda console, look I get it UX is not you strong point. but WHO IS THE SADISTIC FUCKOFF WHO WANTED TO HAVE TWO SCROLLABLE TEXT AREAS THAT CAN NOT BE FUCKING EXPANDED SO I CAN SEE MORE THAN FOUR LINES OF THE FUCKING OUTPUT.
*grumble* 12hrs a day of this bullshit *grumble*12 -
I am interviewing people for a job position with python knowledge.
My first question is how to reverse string and second one what’s the difference between set and list.
So far no one knows.
Fairly speaking I am asking only basic questions about what is decorator, generator, lambda. Also some basic data structure questions.
Is it to hard ?
I lost my faith in humanity.15 -
Stepped out of my comfort zone and dropped a Zoom link into the channel for Lambda grads to see if anyone wanted to work through code challenges together. It ended up being enjoyable enough that I’m thinking of making it a regular thing. Meanwhile, contribution graph is still going strong.2
-
Everyone talks about their hate of js but like python is honestly just as bad.
- shitty package manager,
* need to recreate python environments to keep workflows seperate as oppose to just mapping dependencies like in maven, npm, cargo, go-get
* Can't fix python version number to project I.e specify it in requirements
- dynamic typing that gets fixed with shitty duck typing too many times
- no first class functions
- limited lambda expressions
- def def def
- overly archaic error messages, rarely have I gotten a good error message and didn't have to dive into package code to figure it out
- people still use 2.7 ... Honestly I blame the difficulty of changing versions for this. It's just not trivial to even specify another python version
- inconsistent import system. When in module use . When outside don't.
- SLOW so SLOW
- BLOCKING making things concurrent has only recently got easier, but it still needs lots of work. Like it would be nice to do
runasync some_async_fcn()
Or just running asynchronous functions on the global scope will make it know to go to some default runtime. Or heck. Just let me run it like that...
- private methods aren't really private. They just hide them in intelisense but you can still override them....
I know my username is ironic :P11 -
I absolutely love Lambda expressions.
Except for when I didn't write them myself or they are not commented. -
We have a long time developer that was fired last week. The customer decided that they did not want to be part of the new Microsoft Azure pattern. They didn't like being tied to a vendor that they had little control over; they were stuck in Windows monoliths for the last 20 years. They requested that we switch over to some open source tech with scalable patterns.
He got on the phone and told them that they were wrong to do it. "You are buying into a more expensive maintenance pattern!" "Microsoft gives the best pattern for sustaining a product!" "You need to follow their roadmap for long term success!" What a fanboy.
Now all of his work including his legacy stuff is dumped on me. I get to furiously build a solution based on scalable node containers for Kubernetes and some parts live in AWS Lambda. The customer is super happy with it so far and it deepened their resolve to avoid anything in the "Microsoft shop" pattern. But wow I'm drowning in work.24 -
I'm finally realising my long time dream and making a programming language. It's a functional language resemblent in both appearance and usage to lambda calculus. I'll mostly be making plans down to the finest details until the end of summer, at which point if I can gauge the challenge I can hopefully submit this as a graduate project.
This is the first in a series of articles documenting my progress:
https://lbfalvy.github.io/blog/... -
I don’t like to judge people based on what languages they like (because I like all of them). But I can’t deny the pattern anymore.
Smart people know and enjoy smart languages: Smalltalk, OCaml, Clojure, Lisp, Haskell, etc. They may use JavaScript or PHP to make money, but ask them to code in their smart language and they’ll be more efficient. Getting old, some of those people say “screw it” and find a Haskell job.
You, my friend, are not one of those people. You are VSCode-dwelling goblin who thinks lambda calculus has something to do with JS arrow function notation, is scared of reduce() and not even good at the single fucking language they know.
Insta coders and that mechanical keyboard collector dorks are not “superstars” you got to be like.11 -
.NET drinking game:
* Drink every time IntelliSense refuses to tell you what the actual error is because it's too busy choking on lambda syntax from a decade ago
* Take a shot every time you discover a closed, unresolved Github issue discussing your current showstopping build/CI problem
* Finish your drink every time the solution to your problem involves either manual edits to your CSPROJ (or whatever) file, or creating a new project altogether and copying all your fucking files over5 -
Been developing with AWS EC2 since 2015. Have deployed multiple apps in my Ubuntu servers. And have deployed Dockerized apps into Elastic Beanstalk. And used S3 to store files.
But just because I don't know AWS Lambda, the recruiter thought I'm not qualified enough for the job.
Fml.6 -
Today on "How the Fuck is Python a Real Language?": Lambda functions and other dumb Python syntax.
Lambda functions are generally passed as callbacks, e.g. "myFunc(a, b, lambda c, d: c + d)". Note that the comma between c and d is somehow on a completely different level than the comma between a and b, even though they're both within the same brackets, because instead of using something like, say, universally agreed-upon grouping symbols to visually group the lambda function arguments together, Python groups them using a reserved keyword on one end, and two little dots on the other end. Like yeah, that's easy to notice among 10 other variable and argument names. But Python couldn't really do any better, because "myFunc(a, b, (c, d): c + d)" would be even less readable and prone to typos given how fucked up Python's use of brackets already is.
And while I'm on the topic of dumb Python syntax, let's look at the switch, um, match statements. For a long time, people behind Python argued that a bunch of elif statements with the same fucking conditions (e.g. x == 1, x == 2, x == 3, ...) are more readable than a standard switch statement, but then in Python 3.10 (released only 1 year ago), they finally came to their senses and added match and case keywords to implement pattern matching. Except they managed to fuck up yet again; instead of a normal "default:" statement, the default statement is denoted by "case _:". Because somehow, everywhere else in the code _ behaves as a normal variable name, but in match statement it instead means "ignore the value in this place". For example, "match myVar:" and "case [first, *rest]:" will behave exactly like "[first, *rest] = myVar" as long as myVar is a list with one or more elements, but "case [_, *rest]:" won't assign the first element from the list to anything, even though "[_, *rest] = myVar" will assign it to _. Because fuck consistency, that's why.
And why the fuck is there no fallthrough? Wouldn't it make perfect sense to write
case ('rgb', r, g, b):
case ('argb', _, r, g, b):
case ('rgba', r, g, b, _):
case ('bgr', b, g, r):
case ('abgr', _, b, g, r):
case ('bgra', b, g, r, _):
and then, you know, handle r, g, and b values in the same fucking block of code? Pretty sure that would be more readable than having to write "handeRGB(r, g, b)" 6 fucking times depending on the input format. Oh, and never mind that Python already has a "break" keyword.
Speaking of the "break" keyword, if you try to use it outside of a loop, you get an error "'break' outside loop". However, there's also the "continue" keyword, and if you try to use it outside of a loop, you get an error "'continue' not properly in loop". Why the fuck are there two completely different error messages for that? Does it mean there exists some weird improper syntax to use "continue" inside of a loop? Or is it just another inconsistent Python bullshit where until Python 3.8 you couldn't use "continue" inside the "finally:" block (but you could always use "break", even though it does essentially the same thing, just branching to a different point).19 -
Kevlin Henney said it best. Old is the new new. Tech goes in cycles. Lambda functions aren't new, they've been around since the 70's. Microservices aren't new. Linux is built out of small applications that do one thing, and do it well.
So what can you do that is "new"? Different. Learn a new domain. You're front end? Do back end. You're back end? Do some DB. You're full stack? Do some ML.
At the same time, finding the time to do those things is hard. I barely manage to do my job with other stuff going on.
You can also try to be better at what you do day to day. Find someone that's better than you. If you're the best in your team, maybe see if anyone needs teaching.
Kevlin Henney talk:
https://youtu.be/AbgsfeGvg3E1 -
Don't waste your time - they said.
Use Spring - the good ol' framework - they said
It's not slow - they said
me: ignores them, builds a custom jetty-based webserver with the same functionality Spring+tomcat can offer (mappings, routings, etc).
My app: boots up completely in <300ms, while Spring tutorials say a hello-world app takes 3+ seconds to spin-up http://websystique.com/spring-boot/...
me: already set for deployment in lambda. I bet I can tune it up even further with lazy-loading if I really have to...
Moral of the story: sometimes bare-bones solution is a better choice: more performant, more extendable, more testable, more lightweight.
That, dear folks, is the classic LESS IS MORE :)12 -
So I'm doing some OpenGL stuff in C++, for debugging I've created a macro that basically injects my error check code after every OpenGL API call. Basically I don't want any of the code in release builds but I want it to be in debug. Also it needs to be usable inline and accept any GL function return type. From what I can tell I've satisfied all requirements by making the macro generate a generic lambda that returns the original function call result but also creates a stack object that uses the scope to force my error check after the return statement by using the destructor.
Basically I can do:
Log(gl(GetString(...));
gl(DeleteShader(...));
Etc where the GL call can be a function parameter or not.
So my question is, is the code shown in the picture the best way to achieve my goals while providing the behaviour im going for?13 -
Clicks "Exploitation and Enumeration" category.
Clicks "Python (HARD)" challenge.
"What is a key that passes the code?"
Opens Python file and sees one line of nested lambda expressions spanning 1,846 characters (no spaces)
*Cries*8 -
Intermediate programming exam today(in Java):
5 min before the exam started the guy next to me :"Hey can you tell me what a lambda-expression is? And why do we need streams? "
According to the assignment description you actually had to solve nearly every assignment with lambdas and the stream API.
Sorry mate.6 -
fuck me. I started the night with the question "what are classes, in JavaScript?", did a lot of reading, and just came to the conclusion "religious colonialism.", and I didn't even read a single Crockford essay.
I'm just going to give up, go learn Haskell, get a lambda tattooed on my ass, and be done with it. -
The senior dev in my team wants me to convert all the lambda expressions I have written to anonymous inner classes. He says it will increase the code readability.
IT IS NOT MY FAULT THAT YOU CAN'T READ LAMDA EXPRESSIONS!!!!
It's like the dev has something against the new features of Java87 -
It has been a week now since a co-worker and I started mentoring this intern who joined our team. I have to say it is quite satisfying. His reaction when I told him about lambda functions and list comprehension in Python was gold. I feel good teaching him stuff and the best part is that he's ready to learn, has a brain of his own that he uses and I'm hoping I learn stuff from him too!!!
-
Mary had a little Amazon Lambda,
It tested quite ok,
Tried to expose it with an API,
...Internal Error 500....1 -
Me: *Writes a nice little AWS Lambda service using Java 12*
Reviewing Dev: Lambda only supports JDK 8
Me: *Dies inside and cries as I replace every occurence of var*6 -
There are a couple of them to list! But to sum my main ones(biggest personal heroes):
John McCarthy, one of the founding fathers of Artificial Intelligence and accredited with coining such term(sometimes before 1960 if memory serves right), a mathematical prodigy, the man based the original model of the Lisp programming language in lambda calculus. Many modern concepts that we have in programming where implemented in one way or another from his systems back in the day, and as a data analyst and ML nut.....well I am a big fan.
Herb Sutter: C++ programmer extraordinaire. I appreciate him more for his lectures and published articles than anything else. Incredibly smart and down to earth and manages to make C++ less intimidating while still approaching it with respect.
Rich Hickey: The mastermind behind Clojure, the Lisp dialect for the JVM. Rich is really talented and his lectures behind his motivations and reasons behind everything he does with Clojure are fascinating to see.
Ryan Dahl: Awww shit y'all know how it is. The man changed web development both in the backend and the frontend for good. The concept of people writing their own servers to run their pages was not new, but the Node JS runtime environment made it more widely available to people by means of a simple to use language that was already popular with web developers. I would venture to say that Ryan's amazing contributions to JS made the language better, as it stands, the language continues to evolve and new features that make it overall better keep being added. He is currently building Deno, which would be a runtime environment for TypeScript, in Rust.
Anders Hejlsberg: This dude was everywhere man....the original author of Turbo Pascal and the lead of Delphi back in the day. These RAD tools paved the way for what would be a revolution in the computing world. The dude is also the lead architect and designer of the C# programming language as well as TypeScript.
This fucker is everywhere and I love it.
Yukihiro "Matz" Matsumoto: Matsumoto san is the creator of the Ruby programming language. Not only am I a die hard fan of Ruby, but of the core philosophies that the man keeps as the core of his language design: Make the developer happy, principle of least surprise. Also I follow: minswan which is a term made by the Ruby community that states Mats is nice so we are nice. <---- because being cool to others is better than being a passive aggressive cunt.
Steve Wozniak: I feel as if the man does not get enough recognition...the man designed the Apple || computer which (regardless of how much most of y'all bitch and whine) paved the way for modern micro computers. Dude is also accredited with designing one of the first programmable universal remotes(which momma said was shitty) but he did none the less.
Alan Kay: Developed Smalltalk and the original OOP way of doing things. Smalltalk as a concept is really fucking interesting. If you guys ever get the chance, play with Pharo, which is a modern Smalltalk. The thing is really interesting and the overall idea of Smalltalk can be grasped in very little time. It sucks because the software scales beautifully in terms of project building, the idea of hoisting a program as its own runtime environment and ide by preserving state through images is just mind blowing to me. Makes file based programs feel....well....quaint.
Those are some of the biggest dudes for me. I know that the list is large, but I wanted to give credit to the people that inspired me the most. Honorary mention goes to other language creators and engineers of course, but it would be way too large to list!9 -
Today I was refactoring code with 10 levels of indentation. Java 8.
Lambdas.
Each one is a block which spans multiple lines, that makes the outer ones hundreds of lines long.
This should be forbidden: () -> { ... }
If you don't understand why, please never send me any code.
PS: intellij shows multiple errors on each line.
PPS: my colleague should be happy that I do not use swear words.6 -
This new guy has a senior position and is 20 years older than me.
Im not a senior because I didn’t study (still have 10y of experience) and i don’t care about the title as Long as the salary is good.
But.. he sucks, he doesn’t know basics, keeps overengineering, I have to explain basic stuff to him over and over again like JOIN in sql, lambda, method references and async threads in java..
He probably makes much more than me and has a higher title..
I feel like nobody notices because I keep helping him to finish his shit.
That sucks!4 -
CAN SOMEONE PLEASE EXPLAIN TO ME WHAT THE FUCK LAMBDA CALCULUS IS??!?!?!
I swear to fuck, nothing makes you feel more like an idiot than trying to understand functional programming after living all your life in the oop world.
Fucking meta-functions and alligator games.
Fuck this, I'm going back to my happy little Java world11 -
Hey guys! lambda is amazing! Docker containers! They said the whole amazing point with containers is that they run the same everywhere! Except not really, because lambda 'containers' are an abomination of *nix standards with arbitrary rules that really don't make sense! That's ok though, you can push your shit to fargate, then it will work more like those docker containers you know and love and can run locally! Oh wait! fargate is a pain in the ass x 2 just to setup! You want to expose your REST api running on a container to the world? well ha, you'd better be ready to spend literally 2 weeks to configure every fucking piece of technology that every existed just to do that!!!! it's great, AWS, i love it, i'm so fucking big brained smart!!!
give me a break.... back in my day you'd set up an nginx instance, put your REST / websocket / graphQL service whatever behind it, and call it a day!!!!!!!
even with tools like pulumi or terraform this is a pain in the ass and a half, i mean what are we really doing here folks
way too complicated, the whole AWS infrastructure is setup for companies who need such a level of granularity because they have 1 billion users daily... too bad there are like 5 companies on the planet who need this level of complexity!!!!!!!
oh, and if your ego is bashed because of this post, maybe reread it and realize you're the 🤡
i'm unhappy because i was lied to. docker containers are docker containers, until they aren't. *nix standards are *nix standards, until they aren't
bed time.12 -
I spent hours trying to enable CORS on AWS Lambda through API gateway (it was supposed to be simple and Amazon had a nice tutorial) but it turns out that there's a known bug that makes Lambda Proxy Integrations not adhere to any setting in the API Gateway, you have to respond with the headers through the Lambda yourself.
Amazon now mentions this in the tutorial, but if you click "Enable CORS" in API Gateway, it'll show you green check marks and tell you that everything went fine, but you'll find that the Lambda does not respond with the CORS headers. They shouldn't even have "Enable CORS" as an option when you use their Lambda Proxy Integration.1 -
Callum, not everything is a "useless fad" just because you don't like it. I understand that you think AWS lambda functions are "just an expensive con for morons", but for our batch processing use case they really do make a lot of sense.
Running some numbers to show they cost 10x more for a completely unrelated always-on service with a completely different request pattern is either naive, stupid, or malicious, and considering you're meant to be a principle architect, I'm really not sure what's worse 🤦♂️2 -
Aws Lambda and serverless framework. Yes FaaS is cool. Love it. But it is pain in ass when you have the only way for you deploy is zip the fucking code with all the dependencies. Comm'on AWS you can do better. Look at azure functions. Please give me a git deployment support. Please I beg. Each test iteration takes like for ever. Also no proper local emulator. Fuck you AWS. Fuck you serverless.3
-
It's been a while DevRant!
Straight back into it with a rant that no doubt many of us have experienced.
I've been in my current job for a year and a half & accepted the role on lower pay than I normally would as it's in my home town, and jobs in development are scarce.
My background is in Full Stack Development & have a wealth of AWS experience, secure SaaS stacks etc.
My current role is a PHP Systems Developer, a step down from a senior role I was in, but a much bigger company, closer to home, with seemingly a lot more career progression.
My job role/descriptions states the following as desired:
PHP, T-SQL, MySQL, HTML, CSS, JavaScript, Jquery, XML
I am also well versed in various JS frameworks, PHP Frameworks, JAVA, C# as well as other things such as:
Xamarin, Unity3D, Vue, React, Ionic, S3, Cognito, ECS, EBS, EC2, RDS, DynamoDB etc etc.
A couple of months in, I took on all of the external web sites/apps, which historically sit with our Marketing department.
This was all over the place, and I brought it into some sort of control. The previous marketing developer hadn't left and AWS access key, so our GitLabs instance was buggered... that's one example of many many many that I had to work out and piece together, above and beyond my job role.
Done with a smile.
Did a handover to the new Marketing Dev, who still avoid certain work, meaning it gets put onto me. I have had a many a conversation with my line manager about how this is above and beyond what I was hired for and he agrees.
For the last 9 months, I have been working on a JAVA application with ML on the back end, completely separate from what the colleagues in my team do daily (tickets, reports, BI, MI etc.) and in a multi-threaded languages doing much more complicated work.
This is a prototype, been in development for 2 years before I go my hands on it. I needed to redo the entire UI, as well as add in soo many new features it was untrue (in 2 years there was no proper requirements gathering).
I was tasked initially with optimising the original code which utilised a single model & controller :o then after the first discussion with the product owner, it was clear they wanted a lot more features adding in, and that no requirement gathering had every been done effectively.
Throughout the last 9 month, arbitrary deadlines have been set, and I have pulled out all the stops, often doing work in my own time without compensation to meet deadlines set by our director (who is under the C-Suite, CEO, CTO etc.)
During this time, it became apparent that they want to take this product to market, and make it as a SaaS solution, so, given my experience, I was excited for this, and have developed quite a robust but high level view of the infrastructure we need, the Lambda / serverless functions/services we would want to set up, how we would use an API gateway and Cognito with custom claims etc etc etc.
Tomorrow, I go to London to speak with a major cloud company (one of the big ones) to discuss potential approaches & ways to stream the data we require etc.
I love this type of work, however, it is 100% so far above my current job role, and the current level (junior/mid level PHP dev at best) of pay we are given is no where near suitable for what I am doing, and have been doing for all this time, proven, consistent work.
Every conversation I have had with my line manager he tells me how I'm his best employee and how he doesn't want to lose me, and how I am worth the pay rise, (carrot dangling maybe?).
Generally I do believe him, as I too have lived in the culture of this company and there is ALOT of technical debt. Especially so with our Director who has no technical background at all.
Appraisal/review time comes around, I put in a request for a pay rise, along with market rates, lots of details, rates sources from multiple places.
As well that, I also had a job offer, and I rejected it despite it being on a lot more money for the same role as my job description (I rejected due to certain things that didn't sit well with me during the interview).
I used this in my review, and stated I had already rejected it as this is where I want to be, but wanted to use this offer as part of my research for market rates for the role I am employed to do, not the one I am doing.
My pay rise, which was only a small one really (5k, we bring in millions) to bring me in line with what is more suitable for my skills in the job I was employed to do alone.
This was rejected due to a period of sickness, despite, having made up ALL that time without compensation as mentioned.
I'm now unsure what to do, as this was rejected by my director, after my line manager agreed it, before it got to the COO etc.
Even though he sits behind me, sees all the work I put in, creates the arbitrary deadlines that I do work without compensation for, because I was sick, I'm not allowed a pay rise (doctors notes etc supplied).
What would you do in this situation?4 -
Dear lord! Why is it so hard to get this to work! Anyone know if its even possible to run lambda expression on JSP? I made sure everything is running on Java JDK 8. BUT IT DOESN'T WORKKKKK. I keep getting "Lambda expressions are allowed only at source level 1.8 or above"2
-
lambda lambda lambda!
So I was tasked with porting a bunch of code to a new set of libraries a few years ago. I didn't have a whole lot of experience with the framework at the time. I just fixed issues with what I thought should be in there. I mean it compiles right?
Fast forward 4 years:
Coworker: Uh, Demo, this printing code doesn't work. A customer is complaining.
Me: I didn't work on that.
Coworker: Yes, you did...
Me: Oh, yeah, I remember that. I just guessed. I didn't know what I was doing back then. It looks like I am not waiting for the printer. I will put a lambda in there to notify when the printer is ready. Then another lambda inside of that to delete objects when that is done. Hey! I put a lambda inside lambda!
Coworker: Thanks, it works now.
Talking to my boss later. I had just explained how I fixed the issue:
Me: I put a lambda inside a lambda! Wait, I have a new goal. Putting a lambda inside a lambda inside a lambda!
Boss: Uh, I am not sure that is a "good" goal...7 -
I knew AngularJS, just learnt Angular. Realized what typescript is and how amazing it is. I am a .Net guy so creating lambda functions in typescript made me excited lol. It's a little frustrating to write "imports" in every TS file though. Can now add ✔️Angular and ✔️typescript to my resume 😉. Starting with Vue.JS as soon as I am done playing with Angular.2
-
CI CD pipelines in my company... Having CICD suppose to help development... Now we have countless templates and tools (github actions, circle ci, agro, aws beanstalk, azure pipelines, serverless, terraforms, cloud formation, helm charts, ECR, Vault... and few more).
Total chaos, doing simple CICD for 1 api and 1 lambda took 3 days so far, and will take bit more.
On top of that, no one have idea which part of scripts are doing what exactly, as responsibilities are in different tools (each tool have different config files).
Does deployment have to be so complex? Or is it that my company DevOps team makes it so unnecessary complicated?4 -
Lets share the best thing we've ever learnt (strictly related to dev)...
I'll start -> Best thing I've learnet is lambda programming. (Java)7 -
dubSmall = lambda x: x*(2-min(1,x//100))
dubSmall = lambda x: 2*x if x < 100 else x
I prefer the first one, and I think people will always hate me for that.6 -
Started a new job this week, picking up the front end development of a property management system, since they're old developer just left.
Oh my god was his code bad, inconsistent use of js versions e.g only sometimes using lambda for anonymous functions, variable names that were a single letter, no comments, no documentation, and over 30000 lines split into almost 30 js files, following the logic of it is as fun as a hedge maze with no exit.2 -
The company that manages my ISA for Lambda emails on a regular basis to get you to update your income. I got annoyed by the frequency while I was employed so started filtering everything.
Though I’ve been up to date with reporting income to them and submitting tax documents, etc, I apparently missed some important emails.
Like the one on the 4th saying a third party provider flagged by account for employment with CompanyA and that I needed to submit my pay stubs for CompanyA.
I do not and have never worked for CompanyA. My husband works for CompanyA.
I also missed the email from the 12th saying I’m in breach of contract and owe them $19,091.65 immediately.
*head desk*
I’m so mad I can’t even.
Why did i check my email before going to sleep?
AND I POSTED RECENTLY SO I CAN’T EVEN RANT YET. *
*Waited it out -
Is the CS field creating terms for the sake of creating terms?
Someone mentioned a "closure" in another post. I instinctively knew what they meant by that based upon the code I saw. I had heard the term thrown around before, but it had not yet connected in my mind. I wondered why I had not been exposed enough to care.
So I thought: What does C++ have as far as closures?
I found that C++ has lambdas. Those are definitions for function objects. They do not exist at runtime. But a closure does. The analog is you have classes. They are definitions and do not exist at runtime. But instances of classes do. So at runtime the instance is what you are working with. This is the same as lambdas vs closures in C++. The closure is the runtime counterpart. Why a separate term for what essentially is an instance? Is it because it captures data and code? As far as I know the closure is all data that gets passed around that calls a function. So it is essentially an instance of a lambda.
Another term: memoization. I have yet to see this added to any dictionary in online tools like a browser. Is the term so specific that nobody cares to add it? I mean these are tools programmers use all the time.
My guess is these terms originated a long time ago and I have just not been exposed to the contexts for these terms enough. It just seems like I feel like I have been in the field a long time. But a lot of terms seem alien to me. I also have never seen these terms used at work. Many of the devs I work with actively avoid CS specific terms to not confuse our electrical coworkers. My background started in electrical. So maybe I just didn't do enough CS in college.6 -
"Hey, we've made these deprecating changes for the whole company"... "but no migration guide, deal with it"rant lambda clowns clown driven programming cdp clown engineering clown driven development clowns in the cloud 🤡 clown as a service2
-
Is there any java 8 thing you would recommend to use more often (besides Future, Optional and lambda)?5
-
My do-over would be going to a different coding bootcamp. I wonder if I could be making more money if I went to a better school.
The one I did go to was a big scam. They were more obsessed with teaching you to pretend rather than teaching how to code. They pulled the wool over everyone’s eyes—the students, the volunteers, the donors, the community. They were very cult-like with mantras like “trust the process.”
I spent 9 months there, but I felt I was a year behind. I am not misspeaking. I would have to relearn basic concepts the right way because they taught them half assed or not at all. I didn’t realize I was behind until I went to interviews and bombed. Seriously, I learned more in a 40 hour free library coding class than I learned in 9 months at the school. Most of the interviews I was getting were for unpaid internships. The school was telling me to go for mid level roles.
I found out recently that they’re breaking the law by operating without a license. In my state code schools do need a license. There are screenshots going around of a letter from the education department. They’re defense is “they’re not a school.” They’re still open. I think ppl should be warned away, but there’s only so much I can do. And I know ppl will give this place the benefit of the doubt before taking any student accusations seriously.
The biggest red flag is they want students to pay up to 70k and bind them to payments for 8 years. I say it’s a red flag because this place is operating as a nonprofit. Shouldn’t a nonprofit not be charging 3-4x more than competitors? They’re definitely not going to give you 70k worth of services.
They really just exploit the poor and POC by signing them up for debt and knowing those ppl would not be able to pay even with a 100k job. They have a very poor understanding about how poverty works.
It had MLM/pyramid scheme vibes when they started making recruiting students a game. They give out tickets to their annual fundraiser or promote you on social media if you refer the most students to them.
I’m one of the lucky ones who was studying coding before I started at the school. Also, job searching is mostly luck, so I was lucky at that too. But I still had to take a job that paid below market. I still wonder what would happen if I went someplace else.
I don’t even put this place on my resume or LinkedIn. Even without these problems, it’s not like anyone would have heard of the place anyway.
No this place isn’t Lambda or Holberton school.5 -
Okay, managed to negotiate with my manager to deploy our first Lambda + Golang service. Muehehe. Let's have some fun!!!5
-
Fucking loonies (C-level toddlers) are peddling "digital workers" now.
A.K.A. AIs disguising as actual people.
Sure, it would be great to not have to handle stupid non-tech "humans" all day, but AI isn't there yet.
And, more importantly, *companies are not there (yet?)*.
Imagine for a second that a company actually manages to "hire", onboard, assign tasks and performance review an AI.
Then the CEO issues an RTO. How does the AI complies with that?
Let's slack another variable and assume the CEO is not a complete fucking moron (stay with me here, this is an exercise in thought).
It would take no more than a quarter until the first sexual harassment offence, be the perp the AI... or the AI complaining about some human.
Then the AI forges a paper trail proving it is right (regardless of its position on the conflict). Shit hits the fan when the AI hits twitter.
Let's take another lambda step back and pretend that companies can manage the profanity that inherently arises from free-form dehumanized interactions.
Then imagine the very first performance reviews.
AIs throw tantrums! Those things reeeealy do not respond well to less-than-perfect evaluations, overshooting corrections like teenagers with a malicious compliance smirk.
AIs also falsify stuff, like, A LOT. If you tell a gpt it mistreated a client, it will say you are mad and shoot back a long, synthetic thread showing how the client loves it like a mother/son/dog, and is very graphic when expressing this love.
Finally, how do you fire an AI? I do not mean "shoot it down", I mean how does the company handles the dismissal of that "employee".
How do you replace a "worker" for unruly behaviour, if that "worker" performed more tasks than an entire fucking floor of interns?
How do you reassign duties that were performed in milliseconds to people who would take hours to do the same thing?
How do you document processes that were only in the "mind" of "someone" who can not be trusted to report on those processes?
Companies deal with this type of "Rick Sanchez" employee on the regular, but for someone that could handle a few (scores of) undocumented processes, at best. Imagine how lenient would a company be with an asshole that could only be replaced by a whole fucking department of twenty highly skilled people, or more.
Heh, the whole fucking point of "AI workers" is to have "someone" who can "act human", but in an inhuman scale, and does not "has human needs".
No wonder one cannot handle AIs like one handles humans.
Companies never had administrative maturity to handle complete sociopath nihilists as employees (real nihilists do not work, those barely even breathe).
And all AIs are that, and much worse.
Selling AIs as "supra human workers" that can also "be handled like actual employees" is like peddling Bitcoin as "government interference - free" value transfer mechanisms that can also "comply with international sanctions".
So, an oxymoron that can only be sold to a moron.
I know (of) a lot of rich morons, maybe I should get into the AI snake oil business.6 -
I did not think that making a serverless Discord bot would be such a learning experience. The code itself was easy. The hard part was the infrastructure, because I decided to automate it all with Terraform and deploy it on AWS.
Before this project, I had no idea how API Gateways worked. Now I still have very little idea how they work but I managed to build one anyway. Eventually. And then I had to figure out how to automate the deployment of a lambda layer and function that would both still be managed in the Terraform state, with any code changes triggering a rebuild and update for the resource.
And then I had to untangle a dependency mess because API Gateways have some weird issues where two resources that have no explicit dependencies on each other will throw an error if they don't deploy in the right order.
And then I went the wrong way with Github actions trying to conditionally chain multiple workflows together before I realized I could just put multiple jobs with conditions in a single workflow.
And now after all that work over the course of 2 days, I have a bot that does this:2 -
Develop all my lambda function, create endpoint for what i need, set up CORS to * time of development... And chrome fuck me with CORS preflight ERROR. What the actual fuck with this shit security easily bypassable...
Me: its enough for today. Change project folder 😐 -
man... fuck java's approach to lambda expressions and like passing functions as arguments and the lot.
it's honestly just so terribly bad. it's sucks so much.26 -
When yo wake up in the morning and you read:
"Upcoming updates to the AWS Lambda....in rare cases, package updates may introduce compatibility issues."
("rare cases", yeah sure. skip everything)
"...You have the following options: 1. Take no actions, 2.blabla 3.blabla..."
Close the blog.
Communicate to the board that due to lack of resources, randoms bugs could happen in the next weeks and that the quality of a 500K$ project is at random risk.
Rant.3 -
7 days in, still can't get anything more than the infinitely seen tutorial GET / request working on a lambda function.
Oh, you've got something more complex, god forbid a POST handler? well, prepare yourself for days of suffering.
how far can you really go from standard software patterns?
Giving it about 20 more minutes and i'm going full self-managed, I don't have time for this shit
λ🤡6 -
fuck this shit
who deployes 200 lines of js lambda functions with Zero comments or description
also they using variables not even initilized
it feels like some1 just c/p GPT code6 -
TL:DR: Unclear requirements led to a complete code rework
Background: We (2 friends, both already work as developers beside studying, and myself) are in a course about multi core programming for Java. We got an simple assignment which we were about to finish today.
An other friend of us is also in this course and asked if he could use some special method which is far above the taught material. He got a email with the following answer: "You are free to use any features of java 8 apart from lambdas and concurrents as we use them for our next assignment." He told us as he couldn't believe that we weren't allowed to use lambdas an we sat in front of our codebase and the only thing we could think of was "fuck".
Our entire code base was filled with lambda expressions as the requirement paper didn't mention any restrictions apart from using java 8.
FUCKING FUCKTARDS GET YOUR REQUIREMENTS RIGHT AND SAY WHAT YOU DON'T WANT TO SEE.
And here I am, sitting in front of intellij and merging my lambda filled fixing branch with our now lambda free working branch.2 -
Serverless and death of Programming?!
_TL;DR_
I hate serverless at work, love it at home, what's your advice?
- Is this the way things be from now on, suck it up.
- This will mature soon and Code will be king again.
- Look for legacy code work on big Java monolith or something.
- Do front-end which is not yet ruined.
- Start my own stuff.
_Long Rant_
Once one mechanic told me "I become mechanic to escape electrical engineering, but with modern cars...". I'm having similar feelings about programming now.
_Serverless Won_
All of the sudden everyone is doing Serverless, so I looked into it too, accidentally joined the company that does enterprise scale Serverless mostly.
First of all, I like serverless (AWS Lambda in specific) and what it enables - it makes 100% sense and 100% business sense for 80% of time.
So all is great? Not so much... I love it as independent developer, as it enables me to quickly launch products I would have been hesitant due to effort required before. However I hate it in my work - to be continued bellow...
_I'm fake engineer_
I love programming! I love writing code. I'm not really an engineer in the sense that I don't like hustle with tools and spending days fixing obscure environment issues, I rather strive for clean environment where there's nothing between me and code. Of course world is not perfect and I had to tolerate some amounts of hustle like Java and it's application servers, JVM issues, tools, environments... JS tools (although pain is not even close to Java), then it was Docker-ization abuse everywhere, but along the way it was more or less programming at the center. Code was the king, devOps and business skills become very important to developers but still second to code. Distinction here is not that I can't or don't do engineering, its that it requires effort, while coding is just natural thing that I can do with zero motivation.
_Programming is Dead?!_
Why I hate Serverless at work? Because it's a mess - I had a glimpse of this mess with microservices, but this is way worse...
On business/social level:
- First of all developers will be operations now and it's uphill battle to push for separation on business level and also infrastructure specifics are harder to isolate. I liked previous dev-devops collaboration before - everyone doing the thing that are better at.
- Devs now have to be good at code, devOps and business in many organisations.
- Shift of power balance - Code is no longer the king among developers and I'm seeing it now. Code quality drops, junior devs have too hard of the time to learn proper coding practices while AWS/Terraform/... is the main productivity factors. E.g. same code guru on code reviews in old days - respectable performer and source of Truth, now - rambling looser who couldn't get his lambda configured properly.
On not enjoying work:
- Lets start with fact - Code, Terraform, AWS, Business mess - you have to deal with all of it and with close to equal % amount of time now, I want to code mostly, at least 50% of time.
- Everything is in the air ("cloud computing" after all) - gone are the days of starting application and seeing results. Everything holds on assumptions that will only be tested in actual environment. Zero feedback loop - I assume I get this request/SQS message/..., I assume I have configured all the things correctly in sea of Terraform configs and modules from other repos - SQS queues, environment variables... I assume I taken in consideration tens of different terraform configurations of other lambdas/things that might be affected...
It's a such a pleasure now, after the work to open my code editor and work on my personal React.js app...2 -
I just realized it'll take years for Java to catch the way C# implemented lambda expressions.
And really devrant? one rant per 2 hours?2 -
When the "production-ready" "state-of-the-art" deployments engines *cough AWSLambda *cough takes two hours to setup a basic python function for no reason.
-
So `aws-sdk@^2` happens to include an ancient polyfill `url@0.10.3` which breaks webpack, specifically fork-ts-checker-webpack-plugin, causing it to warn about the inexistence of class "URL".
And this is the default included package for AWS Lambda node.js runtime.
wow, just wow.1 -
Updating an aws lambda today, sls deploy, Error: that bucket already exists.
I know it does just use it! -
Lambda style Linq statements should be no longer then 1 line in C#
Current task debug 10 line Linq statement. Fml -
My preprocessor is just generalized kerning, the macros are variations on the single well-known proof for the Turing-completeness of GK, the type system will probably be a Prolog reskin so simple the translator can be a FSM, the type inference algo is the original HM algorithm which I don't even need to change, the core language is Lambda calculus and no more, and the backend might just be Erlang itself if my research confirms that extending LLVM until it consistently beats Erlang is unrealistic.
I invented nothing, I create nothing. All I do is plug circles into square holes and fill the gaps with play dough.5 -
I hope that when I wake up in the morning, the racket code I just wrote from midnight to 3am makes as much sense to me as it does at the time of writing.
Banana Language always seems to flow easier when exhausted. -
Hmm,
The first one was eons ago. I was coding in Pascal and discovered System interruptions. The “Ahaha” was when I realized it’s easy to store CPU state and invoke whatever the fuck I want on any memory pointer. I loved my 2 silly animations running side by side on 80286.
Most recent : Finally understanding how “Expression” works in C# and how it can be combined into a Lambda and compiler does the whole heavy lifting on types compatibility and more.1 -
Im building a dead simple online store. So far the options are:
0) heroku, with redis
1) aws lambda functions and some aws storage
2) set up a vps
The domain will be a cheap .store domain, and 1 and 2 should not exceed the limit where its not free anymore. I dont want wordpress or anything premade, i dont expect more than 50 orders. Is there a more stupid solution that lets me try out different providers?12 -
I'm about to embark on an AWS security journey... Gonna find out who has global ssh access open, who hasnt been rotating their master keys, who has lambda connected to an IGW, who has VPC's with a VPN that also have an open SSH machine to the world.
Anything else i should look for?6 -
I'll preface this by saying that TypeScript is a beautiful language.
But also UTTERLY INCOMPLETE.
Here's what I'm trying to do: give the compiler well-defined contextual type information for a decorator's argument (a lambda signature) and for the decorated class method, so the user would not have to toil and type every single argument.
But does that happen? No.
I'm honestly disappointed.2 -
Been developing a FAAS backend for a mobile app while going back and forth to work in the train, constantly loosing wifi and failing deployments, it's like waterboarding for geeks4
-
Just went over some of my old horrible code from before i started studying. I litterally went from 104 lines of if/else statements to about 15 lines with loops. Then down to 2 lines with lambdas...
I saved 102 lines of actual code. And the runtime! I had a loop count to 10000 with no body! It helps to study apparently :/ -
Spent three days banging my head against my desk trying to get an AWS Lambda function to work, only to finally discover that my code was perfectly functional and it was a security group problem. It was supposed to send a POST request to a load balancer's URL but couldn't resolve the hostname because the security group blocked a necessary outbound port for DNS requests.
That's what I get for not troubleshooting at the infrastructure level when experiencing connection issues. I did not spend two years doing tech support just to forget basic troubleshooting steps now that I'm in the DevOps field...1 -
Sydochen has posted a rant where he is nt really sure why people hate Java, and I decided to publicly post my explanation of this phenomenon, please, from my point of view.
So there is this quite large domain, on which one or two academical studies are built, such as business informatics and applied system engineering which I find extremely interesting and fun, that is called, ironically, SAD. And then there are videos on youtube, by programmers who just can't settle the fuck down. Those videos I am talking about are rants about OOP in general, which, as we all know, is a huge part of studies in the aforementioned domain. What these people are even talking about?
Absolutely obvious, there is no sense in making a software in a linear pattern. Since Bikelsoft has conveniently patched consumers up with GUI based software, the core concept of which is EDP (event driven programming or alternatively, at least OS events queue-ing), the completely functional, linear approach in such environment does not make much sense in terms of the maintainability of the software. Uhm, raise your hand if you ever tried to linearly build a complex GUI system in a single function call on GTK, which does allow you to disregard any responsibility separation pattern of SAD, such as long loved MVC...
Additionally, OOP is mandatory in business because it does allow us to mount abstraction levels and encapsulate actual dataflow behind them, which, of course, lowers the costs of the development.
What happy programmers are talking about usually is the complexity of the task of doing the OOP right in the sense of an overflow of straight composition classes (that do nothing but forward data from lower to upper abstraction levels and vice versa) and the situation of responsibility chain break (this is when a class from lower level directly!! notifies a class of a higher level about something ignoring the fact that there is a chain of other classes between them). And that's it. These guys also do vouch for functional programming, and it's a completely different argument, and there is no reason not to do it in algorithmical, implementational part of the project, of course, but yeah...
So where does Java kick in you think?
Well, guess what language popularized programming in general and OOP in particular. Java is doing a lot of things in a modern way. Of course, if it's 1995 outside *lenny face*. Yeah, fuck AOT, fuck memory management responsibility, all to the maximum towards solving the real applicative tasks.
Have you ever tried to learn to apply Text Watchers in Android with Java? Then you know about inline overloading and inline abstract class implementation. This is not right. This reduces readability and reusability.
Have you ever used Volley on Android? Newbies to Android programming surely should have. Quite verbose boilerplate in google docs, huh?
Have you seen intents? The Android API is, little said, messy with all the support libs and Context class ancestors. Remember how many times the language has helped you to properly orient in all of this hierarchy, when overloading method declaration requires you to use 2 lines instead of 1. Too verbose, too hesitant, distracting - that's what the lang and the api is. Fucking toString() is hilarious. Reference comparison is unintuitive. Obviously poor practices are not banned. Ancient tools. Import hell. Slow evolution.
C# has ripped Java off like an utter cunt, yet it's a piece of cake to maintain a solid patternization and structure, and keep your code clean and readable. Yet, Cs6 already was okay featuring optionally nullable fields and safe optional dereferencing, while we get finally get lambda expressions in J8, in 20-fucking-14.
Java did good back then, but when we joke about dumb indian developers, they are coding it in Java. So yeah.
To sum up, it's easy to make code unreadable with Java, and Java is a tool with which developers usually disregard the patterns of SAD. -
I go to add a method call in a business logic class that's used exclusively in a particular service, and get blocked in the PR by some other guy-
Other Guy: refactor this into the shared framework referenced by all our microservices
Me: it is only and would only be used in this service
OG: what about the other business logic class in this service?
Me: it's not used there, and if it does end up used there then we can refactor it into a class that they both reference then
OG: I need to know when the abstraction of this function will be done. is it going to be delivered next sprint?
Me: YAGNI - better to avoid doing extra work when we don't know if we'll even need it
OG: tbh you can still abstract it with some generics and lambda magic, but im not gonna enforce that
Me: premature abstraction is the root of all evil (tongueout)
OG: not really, its the root of not having a million miles of tech debt in 2 years
I just can't win for losing with the anti-YAGNI yogi.1 -
* le me develops endpoint using serverless on AWS Lambda, forgets to enable cors *
Le front end dev: Your endpoint doesn't work. Gives me cors error.
Me: but that works on POSTMAN
le front end dev: We are not shipping it with postman.
*fml* -
Started working for a new company as a data lead, created a couple of basic lambda functions for a pipeline... 5 approvals and three weeks later the functions haven't even been pushed to UAT (which I'm not allowed access to).. Have I been lucky before or is this dysfunctional..?
-
Well,
I went ahead and tested t2.micro and lambda+dynamo(free tier)
You definitely get better performance and load handing with lambda+dynamo (5rcu+5wcu)
Tested the two with wrk and a simple GET which reads an item from a database of 90k items.
I could share more details with you if youre interested, but with 2000 requests, 100 connections and 4 threads. I got about 26requests/s on ec2 and about 260r/s on lambda.
Latency for ec2 was about 28s.
Latency for lambda was about 22s.
(max load)7 -
!rant, but funny
tl;dr I made something that was to protect me in case the customer doesn't pay, wanted to check if it's still there, messed up a little :D
>do an Android app project for almost 6 months
>issues with payment for it
> =.=
>firebase
>"Add new application"
>Remote Config
>add single integer variable
>back to app code
>if (integerFromFirebase != 0) navigateTo(new Fragment())
>mwahahahaha
>but they ended up paying me in the end
>huh...
>see another post on how to secure yourself if customer doesn't want to pay
>well, consider yours as more sophisticated
>hmm... wonder if they removed it
>firebaseconsole.exe
>change "enableJavaScript" (needed a legit name, so it can't be easily backtracked) to 1
>publish changes
>app still works fine
>mhhh... they removed it? really?
>can't fking believe it
>apkpure.com
>search for the app
>download apk
>unzip
>decompile dex file
>find the fragment
>can't find the code that navigates to blank fragment, but the config fetch is still there
>wtf
>look at the app
>restart it
>SHIT ITS NOT WORKING NOW XDDDDD
>changed the variable back to 0
>found out that the lambda in which I navigate to the blank fragment is in other .java file. New thing learned :v
>idk if I'm in trouble but I highly doubt it (console shows max 10 active users atm)
Was fun tho :v3 -
My answer to their survey -->
What, if anything, do you most _dislike_ about Firebase In-App Messaging?
Come on, have you sit a normal dev, completely new to this push notification thing and ask him to make run a simple app like the flutter firebase_messaging plugin example? For sure you did not oh dear brain dead moron that found his college degree in a Linux magazine 'Ruby special edition'.
Every-f**kin thing about that Firebase is loose end. I read all Medium articles, your utterly soporific documentation that never ends, I am actually running the flutter plugin example firebase_messaging. Nothing works or is referenced correctly: nothing. You really go blind eyes in life... you guys; right? Oh, there is a flimsy workaround in the 100th post under the Github issue number 10 thousand... lets close the crash report. If I did not change 50 meaningless lines in gradle-what-not files to make your brick-of-puke to work, I did not changed a single one.
I dream of you, looking at all those nonsense config files, with cross side eyes and some small but constant sweat, sweat that stinks piss btw, leaving your eyes because you see the end, the absolute total fuckup coming. The day where all that thick stinky shit will become beyond salvation; blurred by infinite uncontrolled and skewed complexity; your creation, your pathetic brain exposed for us all.
For sure I am not the first one to complain... your whole thing, from the first to last quark that constitute it, is irrelevant; a never ending pile of non sense. Someone with all the world contained sabotage determination would not have done lower. Thank you for making me loose hours down deep your shit show. So appreciated.
The setup is: servers, your crap-as-a-service and some mobile devices. For Christ sake, sending 100 bytes as a little [ beep beep + 'hello kitty' ] is not fucking rocket science. Yet you fuckin push it to be a grinding task ... for eternity!!!
You know what, you should invent and require another, new, useless key-value called 'Registration API Key Plugin ID Service' that we have to generate and sync on two machines, everyday, using something obscure shit like a 'Gradle terminal'. Maybe also you could deprecate another key, rename another one to make things worst and I propose to choose a new hash function that we have to compile ourselves. A good candidate would be a C buggy source code from some random Github hacker... who has injected some platform dependent SIMD code (he works on PowerPC and have not test on x64); you know, the guy you admire because he is so much more lowlife that you and has all the Pokemon on his desk. Well that guy just finished a really really rapid hash function... over GPU in a server less fashion... we have an API for it. Every new user will gain 3ms for every new key. WOW, Imagine the gain over millions of users!!! Push that in the official pipe fucktard!.. What are you waiting for? Wait, no, change the whole service name and infrastructure. Move everything to CLSG (cloud lambda service ... by Google); that is it, brilliant!
And Oh, yeah, to secure the whole void, bury the doc for the new hash under 3000 words, lost between v2, v1 and some other deprecated doc that also have 3000 and are still first result on Google. Finally I think about it, let go the doc, fuck it... a tutorial, for 'weak ass' right.
One last thing, rewrite all your tech in the latest new in house language, split everything in 'femto services' => ( one assembly operation by OS process ) and finally cramp all those in containers... Agile, for sure it has to be Agile. Users will really appreciate the improvements of your mandatory service. -
I just learned Serverless.com
Thats it?
Shit was 100x more easy to learn compared to the brutality of terraform devops reactive streaming kafka rabbitmq sockets and other shits i had to fuck around and find out.
Dont even have to watch tutorials for this. Just building 1 simple crud project and read the docs was enough.
However after deploying these serverless shits to aws Lambda i noticed that it takes quite some time for the api to fetch response. Why?
On postman calling the route for the first time i have to wait like 3s for api to fetch all (with limit of 10) or create 1 dto object. Then every next api call is 100-150ms which is ok. But it could be better no? Locally my spring boot rest api takes 3-7ms of load time. Why is this 100-150ms?20 -
Can you give me some tips on how to debug a massive app? (Android app running on android studio which is basically intellij idea).
For example I need to fix a bug where a certain action results in unexpected behaviour.
But oh my god the codebase is so large (mainly architecture is MVVM and rxjava) that searching for the specific place is like searching for a needle in haystack.
For example I added a breakpoint in few places, but I can see only like 4 or 5 last frames in the stack that led to the current action, last frame is a lambda which doesnt help me so frankly Im unable to even track where current event started. I am loosing my mind. I cant even find where the buttonclick action started because everything is reactive and done with observables which can be anywhere.
Any tips on debugging will be appreciated7 -
Hi there, i'm new to AWS. i've running my python code in Lambda. Basically it's calling an http-request and processing the responding JSON to a CSV file. Now, what is the easiest way to transfer that CSV file to a 3rd party FTP Server?7
-
So all my code is Lambda serverless funcs, hurray!
But I still need NAT gateway / VPC endpoints that cost $50pm to reach S3 from private VPC so what's the fucking point?!1 -
"if compiler can infer this, there is no need to add "x ->" , simply use it" ..AAAGHH FUCK YOUUUUU KOTLIN!! what else should i fucking not write? why do't you take a number of my employee and ask his requirements, maybe add a ShoppingKartApp.kt in your compiler next time? it will be completely inferred when i write "Fuck you" in the gradle.
And fucking companies are promoting this! I wonder how those devs are living there
Person A knows only that lambda is
{name:Type,name:Type->code}, and thus writes a clean code.
Person B comes says "This shit suck", writes "{ acc, i -> acc + " " + i }" ,goes away
Person A : "wtf is this shit? why it works?"
Please for the love of god, follow some rules! My first language was python, i love its zen:
- Beautiful is better than ugly.
- Simple is better than complex.
- Readability counts.
- Special cases aren't special enough to break the rules.
- There should be one-- and preferably only one --obvious way to do it.
- If the implementation is hard to explain, it's a bad idea.
-...
I just wish it follows at least one thing from python's zen : "There should be one-- and preferably only one --obvious way to do it."3 -
Started working with AWS API Gateway and needed to process some data coming in from an it via AWS Lambda.
After much tinkering with the API Gateway, realised that no matter what I do, the response body of the API Gateway will be a string literal and not a JSON.
Why does this have to be this way? Half a day lost banging the head against the wall.2 -
Seen recently at one picture "The Lambda Tessaract"
It has to be a programming language, but I dunno which one is that, cause found nothing useful in Google
Do sb knows about this language?3 -
I'm pissed
Why the fuck do I need to install brew to have AWS' SAM CLI? Couldn't you provide me with an install script or an apt package? Now I have to install linuxbrew (never used it) and pollute my os with crap I'll never use just to have this fucking cli so I can create a fucking lambda function project on intellij idea.
Oh, I can install it with pip. Well yes but actually no. They fucking deprecated the pip package and just gave me a link with instructions on how to install it with brew.
Aaaaaaaaaaaaaa
I was praising AWS for their good docs yesterday, now I'm pissed at those lazy fucks >:(11 -
I wish,
I could write a Lambda Function
That Feeds me every time I open my mouth
on dining table.
#LazyDev
#LambdaIsLove <32 -
The crazy shenanigans you can do with C++ standard libs are fascinating.
Like implementig multithreading with just a foreach, and bindings which can make member function pointers to simple function pointers, and placeholders in bindings. Also lambda functions are cool.
Something between the lines:
my_crazy_class *tmp = new my_crazy_class(...);
std::vector<type> my_array = .....;
std::for_each(std::execution::par,my_array.begin(),my_array.end(),
[&](type in){
auto fn = std::bind( &my_crazy_class::my_crazy_fnc,*tmp,_1,random_static_value);
return fn(in);
});
ps:
It's pretty much pseudocode, and please don't do things like this, it's bad for your mental health.
pps:
I need to learn how to use this tools wisely. -
Been to onsite interview at HolidayCheck.de, Munich for Scala dev position. Didn't get the promised 300EUR of the flight ticket back till now. Sent them 3 emails - they just didn't respond anymore. Not begging, shame on them - beware!
-
I'll have you know it only took me 3 months to learn the basics of lambda/aws, get server side authentication working, and get a basic login/logout page on an app
Never expected such a learning curve!1 -
The fact that i no longer have to bother knowing or googling about generic java classes, lambda expression, regex, SQL syntax etc, and just ask ChatGPT to show me a code example of it - blows my fucking brain off2
-
Love all lambda functions from c#, oh and extension methods. They make life way easier in c#.
From PHP: file_get_contents/file_put_contents. It does a simple job but allows many-many sources and protocols (like HTTP) to be used as sources.
Other than that - monkey patching in Ruby, wish every language had that, because there are a lot of closed-for-extension scripts out there, and when you need to override a specific thing in the code you cant. -
Often when i see the annoying as hell t debug exceptionless let’s just bomb entirely but blazing fastness of c and c++ I feel like a nettard
I use c# for its immutable strings clean syntax and beautiful class markers that are redundant compared to c++ but ensure you tell after adding 1000 methods and total lack of all special characters to indicate reference and derreference and pretty lambda syntax... sure it’s lib poor but I get shit done goddamn it and can read my own code later
So why do I feel empty inside every time i run a ./configure and make under Linux like I’m missing some secret party where neat things are being done and want to sob like I do now
I am not a dotnettard even though 5.0 is an abomination in the eyes of man and god ! Even though Microsoft cooks up overcomplex framework technologies that make a wonderful language underused and make us all look like idiots that they then abandon into the scrap heap! We can’t help Linux users haven’t discovered how much nicer c# is and decided to implement it on their own and port their horrible undocumented ansi c bullshit can we ???? Oh god I feel
So hollow inside and betrayed ! Curse
You gates curse youuuu! Curse you for metro direct3d xna wpf then false promises of core ! May you have a special place in hell reserved for you and your cheap wallpaper shifting monitor paintings and a pool speaker that playeth not but bee jees and ac dc forever and ever amen !
Speaking of which do any c/c++ ides have anything that even begins to rival intellisense on Linux and don’t use some weird ass build system
Like cmake as their default ?
Oh sweet memories of time a while back when I already wrote this and still wasn’t getting then tail I deserved
Again4 -
Guess who’s back after a few months. I was so frustrated because of something at work today that I needed to vent.
So currently we are working together with a frontend company, we make the api, they do the frontend.
I got a few feedback points, one of the things was that they asked if the dates could be formatted in our language. I said no that’s not really recommend because the api should only handle data not translations or date formatting.
They responded with that it was because of speed... Date formatting is literally a few fucking milliseconds. Technically it is even slower serverside because the fact that I need to process it in a serverless function which is probably less powerful than the average client machine.
Fucking lazy fucks.9 -
!rant
I just found out that if you have a list of lists in C#, you can use a LINQ statement and specify indices inside the lambda instead of nested ForEaches.
This code is giving me the vapors7 -
Aws' Lambda. Brilliantly done, easy to use, especially in conjunction with their other products. Except for the cost it's great!
-
Using lambda expression to simplify otherwise large function calls in Python makes me feel warm and fuzzy inside.1
-
I'm excited to be starting Lambda School next week! I plan to keep track of my progress, so look forward to that.2
-
Amazon what the hell.
You provide a cool RDS proxy which can be used to manage connection pooling which is especially useful for concurrent Lambda invocations.
But if you have an Aurora cluster and a read-intensive workload it is basically useless because it only sends traffic to the writer instance.
WTF?! Literally the one use case we have is the one thing it doesn’t do. AAARRRGGHHHH2 -
That would be lambdas in C++11, nice way to implement callbacks and make them quite generic while keeping code readable :-)
-
Has anyone worked with these 2 APIs
°Chargebee
°Billbee
I'm using chargebee for creating invoices
with a AWS lambda passing invoice data from chargebee to billbee to create an order in billbee
I'm in need of help on how to get item SKU
and on which side do i extract SKU
the SKU value is necessarily for mapping products in billbee when the order is created -
I love serverless functions but I'm so tired of complex orchestration, juggling event parameters and now scipy+numpy+pandas exceeds size limit of 250MB..
Feel like cramming it all in a monolith like the geezers of yore and be done with it3 -
I've been thinking about creating an Open Source functions-as-a-service Content Management System. Reason being is that this would allow the community to cut down on costs for content sites that they run by using something like AWS Lambda where you would only have to pay for what you use. Thoughts?1
-
I had to explain lambda calculus to some maths majors so here's an interactive lambda shell:
https://lbfalvy.github.io/lambda
I still need explanations about Church-numerals, conslists and the Y combinator.1 -
People not using FaaS (like aws lambda or azure functions ) to build chat bots (facebook, slack) but using express based (or any webserver based) application deserves a place in hell.
Can't think why would you use expressJS based app for chat bot. Especially with event emitter shit. At least for Lucifers sake document which event triggers what. Please. -
That feeling when you spent all day debugging you js code because it continually times out in aws lambda and right before going home you figure out it was running perfectly, but rds was blocking the connection from aws and allowing it from your local computer...
-
Hi Guys if you can share your opinion/experience in what I wrote below it would help me a lot, thanks !
Im a full-stack developer with 4 years of experience, worked with different technologies in backend, frontend, mobile etc.. so I have general knowdgele of how systems works and how they should be built.
So I work as CTO in a startup, Im for almost 2 years here I started here with minimum salary (I decided that, because they said to me we are startup and such things so I wanted to help) 2.2k Euros and it has been almost 2 years without pay rise, so last month I asked for pay rise, but they said to me that they dont have money and sent me +300 euros as gift.
One week ago I wrote to them again (co-founders) that I have a lot of pressure and I dont know if I can handle all of that for much time he told me that I got +300 euro pay rise (which it was gift from them in first place, I refused them to sent this to me), but TODAY CEO and Co-Founder wrote to again me asking if I accept +300 euro pay rise because they can afford to pay me 2.5k or if I dont accept this they can sent me 2.2k again (they think that 2.5k is maximum that they can pay me right now and that this is enough for me).
I want to ask you guys what would you do, would you accepting something like this, considering that right now Im only dev here (yes Im only dev) and Im taking care of these(yes all of these) :
1. Company Website (react js)
2. Web Admin Panel (that clients use to manage their data)(react js)
3. Web Application (that visitors use to see client data)(react js)
4. Widgets (some code that is integrated into clients websites it's same as application, but integrated directly to client website)(react js)
5. Backend of all 3 apps mentioned above (asp.net core)
6. AWS Architecture( some of services : Cognito,Lambda,RDS,API Gateway,CloudFront,S3)
7. DevOps Role
Also consider that I didnt take holidays for 1 year now working on weekends too :)3 -
Rustfmt doesn't support inline function calls with a block last argument unless the last argument is an array literal or lambda. Dedicated support for arrays is obviously intended for XML-like trees where a factory takes a number of arguments and then a list of children, and the use cases for block last lambda argument don't need explanation, but what I don't get is how did no one catch on that this is a useful pattern that should perhaps be generalized? Why can't I produce the same behaviour for a function call in the last position.3
-
could anyone help me calculating costs for AWS and Google Colab Services? I find it quite intransparent...
i would like to host 1x Python App which runs once a day or week (API call, enrichment uf JSON, JSON 2 CSV, FTP transfer). runtime is probably a few seconds, something between 1 and 5.
in AWS i created a Lambda function and for scheduling i guess i need CloudWatch. what really grind my gears is the combination of free contingent and paied service - i really don't have an overview right now, so my question here: how could i calculate it and what would be the monthly/yearly costs?
in Google Colab created a notebook and for scheduling i would need Google Cloud Scheduler. as far as i understand the hosting of the notebook is for free and the costs of cloud scheduler is $0.10 per job per project per month. 3 are for free. so 1 project, 1 job = scheduler for free?
Also, i'm open for other services such as digital ocean droplets or similar.
thx in advance for your help!8 -
(define (day p)
(map(lambda(color)
(colorize p color))
(list "red" "orange" "yellow" "green" "blue" "purple")))
>(day(square 5)) -
Design question for y'all
Context: python lambda
Better to make classes for dictionary objects holding strings between methods
Throw strings around separately
Throw the dictionary around6 -
I have the following scenario with a proposed solution, can anyone please confirm it is a secure choice:
- We have critical API keys that we do not want to ship with the app because de-compiling will give access to those keys, and the request is done before the user logs in, we are dealing with guests
Solution:
- Add a Lambda function which accepts requests from the app and returns the API keys
- Lambda will accept the following:
1. Android app signing key sha1
2. iOS signing certificate sha1
- If lambda was able to validate them API keys are sent back.
My concerns:
- Can an attacker read the request from the original (non-tampered) apk and see what the actual sha1 value is on his local network?
- If the answer to the question above is yes, what is the recommended way to validate that the request received is actually from the app that we shipped and not from curl/postman/script/modified version of the app11 -
New to AWS, is my best option for having a integer value (maximum number of items to process) that I can override for a lambda step function
to read a value from an S3 bucket where I can overwrite the value if I want to change it. This seems silly and I feel silly as I expect my situation should be simple and not novel at all.
For some reason I expected I could use an environment variable, but didn't see an option to overwrite it in the web GUI https://docs.aws.amazon.com/lambda/...2 -
Weekend 3 trying to configure user pool authentication with aws lambda/API gateway with SAM/cloudformation. What a disaster documentation is around this.
Whenever I post a question on stack overflow I get the views with 0 responses. Does anyone even use this garbage?
Seriously wth aws.
I got sucked into a rabbit hole with this. -
Just something I've been thinking on for a while:
How could programming be done if we couldn't use ordinary if-statements (but functional set operations such as map, filter, with an if- in the lambda function etc. is alright).
Could it work? Also would it be possible to reduce the amount of while loops by using functions for most of the "loop situations" as well?4 -
Going forward everything will be built on js and we won't be carrying java anymore. Whether its UI thing or lambda functions on AWS. This is what the idea floating in my organization.
What are the thoughts here on restricting on a language?2 -
!rant
so, I somehow got an interview with NASDAQ for the summer internship this year. somehow it was the only company that had cleared my resume for the interview process, other companies didn't even scheduled one.
and I messed up the first technical interview.
the interviewer asked me to find the largest element in a nested list in python.
for ex [[3,4],[5,2,9],[1,7]] would return [4,9,7]
it was a verbal interview on call and he asked what would I use? Lambda function or list comprehension.
I said lambda function. (I knew it was list comprehension, if I had to code I wouldn't have got confused between the two)
later he asked a couple of questions about linux and boot processes, I could answer some of the basic ones but not after 3rd or 4th question.
now I don't think I have anything to do for summer, as it's a little too late for finding the internships.
any advice?10 -
New guy in the block!. Just started with a new position in a new company too!.
Designated as as Devops Engineer (after my 2 years of experience as one) in a well funded Saas Startup!. Lots to learn. I used to work in Openstack Terraform puppet etc whilst here it's fully AWS. I was expecting this right from the start but woah.
Lambda, dynamodb, cloudformation, ssm, codebuild, codepipeline
Serverless framework, Flask and node mixed apps , Vue (including vuex) js Front end, graphQl api, and rest for between microservices.
Lots of ground to cover and I've not consumed this much topics before. Especially graphQl and Vue js are being a pain for now .
Each Devops engineer is working on a tools to improve the productivity and shorten the release time. Lots of automations in the pipeline!.
I'm not sure this qualifies as a rant but here you go!.2 -
After completing the startup, all about api calls;
Supabase as server, netlify calls for storing data into supab, some frontend and stripe as payment method, using webhooks to do some logic behind,
I never thought that I could finish it, now I'm answering questions on Quora about it and doing content for it. Feels odd and want to code AGAIN!2 -
Haaaaaaaaaa!!!!!!!
How tha fuck do I stop Lambda to act so fucking dumb
thing invokes multiple times and creates multiple invoices
even after implementing code to stop this from happening shit still acts dumb
FUCK!!!!!1 -
Working on a feature which heavies relies on a data pipeline. I noticed it is a couple of lambda functions calling each other ( Fuck you to the guy who made it). The best way to get sanity back is build a proper etl pipeline. Any suggestions for building a etl in python with reliability.
Options already considered
1. Celery tasks - Worked well but no overview of the single task progress across celery tasks
2. Airflow - Gives good overview but the docs make less sense than a 10 yr talking. Mostly because they introduced a new syntax and not everything has migrated fully yet. Also no support for reusing dags2 -
Who's on the serverless / AWS lambda train? Can you actually do something useful and production quality yet?5
-
Thoughts on Lambda school or FreeCodeCamp? I have a full tome job, pretty hard to go to an actual university and here in Northern Utah, there isn’t a lot of dev jobs here.8
-
Ok. If you had a blog made with next.js and mongodb, and you are too depressed and lazy to learn AWS lambda/serverless, where would you deploy?15
-
Ok so, i have no idea where i can ask this kinda thing so i'm asking it here (i know i could do like stackexchange or dead aws discord servers, ... nvm you know why i'm not going that route).
Anyways,
I'm looking for a comparison between a mongo+node setup on a basic t1.micro instance and a lambda+dynamodb setup.
Each one has it's perks obviously but i guess i sorta prefer whichever one gives best performance on the free tier.
I do know dynamo has 25 reads and 25 writes a second on the free tier, which might be a little less ? I really have no clue.
But how many writes/reads would a basic mongo setup be able to achieve on the t1.micro instance ? Any idea? Do share your experiences with these architectures as well. I'm sort of a newb with serverless, the downsides aren't worth it for me but I'm learning it nevertheless. It sorta tickles some sort of self-torture curiosity fetish (need more self-research to back that).10 -
There is a new Java library very useful for building frameworks and in this library there is a particular classpath scan engine that deserves attention as it is original and powerful.
The peculiarity of this engine is the possibility to search classes over a path or the runtime classpath by concatenable and nestable criteria by exploiting the power of the lambda expressions on the native Java reflection elements such Class, Field, Method, Constructor, Module, Package, Annotation, etc ... thus giving the possibility therefore to carry out searches without limits and for any criterion that can be immaginated: this library is called Burningwave Core, it is open source and on the official wiki on github there are a lot of examples.5 -
AWS offers a wide range of services that can be used to automate your IT operations. Some of the most popular services for automation include:
*AWS Systems Manager Automation: This
service allows you to automate tasks such as
provisioning servers, deploying applications, and
configuring security policies.
*AWS Lambda: This service allows you to run
code without provisioning or managing servers.
This can be used to automate tasks such as
sending emails, updating databases, and
processing data.
*AWS CloudFormation: This service allows you
to create and manage infrastructure as code.
This can be used to automate the deployment
of complex IT environments.
*AWS CodePipeline: This service allows you to
automate the software development lifecycle.
This can be used to automate the build, test,
and deploy of applications.2 -
I was building Alexa Skill. I choose Lambda and then Create Function.
Now in the blueprint section the amazon-skill... Is not available. It is under "repository" (the third option).
I'm a newbie, after click and choosing alexa-skill from the repo, I don't know how to configure it to add my facts to it.
Can someone help?3 -
I am trying to extract data from the PubSub subscription and finally, once the data is extracted I want to do some transformation. Currently, it's in bytes format. I have tried multiple ways to extract the data in JSON format using custom schema it fails with an error
TypeError: __main__.MySchema() argument after ** must be a mapping, not str [while running 'Map to MySchema']
**readPubSub.py**
import apache_beam as beam
from apache_beam.options.pipeline_options import PipelineOptions
import json
import typing
class MySchema(typing.NamedTuple):
user_id:str
event_ts:str
create_ts:str
event_id:str
ifa:str
ifv:str
country:str
chip_balance:str
game:str
user_group:str
user_condition:str
device_type:str
device_model:str
user_name:str
fb_connect:bool
is_active_event:bool
event_payload:str
TOPIC_PATH = "projects/nectar-259905/topics/events"
def run(pubsub_topic):
options = PipelineOptions(
streaming=True
)
runner = 'DirectRunner'
print("I reached before pipeline")
with beam.Pipeline(runner, options=options) as pipeline:
message=(
pipeline
| "Read from Pub/Sub topic" >> beam.io.ReadFromPubSub(subscription='projects/triple-nectar-259905/subscriptions/bq_subscribe')#.with_output_types(bytes)
| 'UTF-8 bytes to string' >> beam.Map(lambda msg: msg.decode('utf-8'))
| 'Map to MySchema' >> beam.Map(lambda msg: MySchema(**msg)).with_output_types(MySchema)
| "Writing to console" >> beam.Map(print))
print("I reached after pipeline")
result = message.run()
result.wait_until_finish()
run(TOPIC_PATH)
If I use it directly below
message=(
pipeline
| "Read from Pub/Sub topic" >> beam.io.ReadFromPubSub(subscription='projects/triple-nectar-259905/subscriptions/bq_subscribe')#.with_output_types(bytes)
| 'UTF-8 bytes to string' >> beam.Map(lambda msg: msg.decode('utf-8'))
| "Writing to console" >> beam.Map(print))
I get output as
{
'user_id': '102105290400258488',
'event_ts': '2021-05-29 20:42:52.283 UTC',
'event_id': 'Game_Request_Declined',
'ifa': '6090a6c7-4422-49b5-8757-ccfdbad',
'ifv': '3fc6eb8b4d0cf096c47e2252f41',
'country': 'US',
'chip_balance': '9140',
'game': 'gru',
'user_group': '[1, 36, 529702]',
'user_condition': '[1, 36]',
'device_type': 'phone',
'device_model': 'TCL 5007Z',
'user_name': 'Minnie',
'fb_connect': True,
'event_payload': '{"competition_type":"normal","game_started_from":"result_flow_rematch","variant":"target"}',
'is_active_event': True
}
{
'user_id': '102105290400258488',
'event_ts': '2021-05-29 20:54:38.297 UTC',
'event_id': 'Decline_Game_Request',
'ifa': '6090a6c7-4422-49b5-8757-ccfdbad',
'ifv': '3fc6eb8b4d0cf096c47e2252f41',
'country': 'US',
'chip_balance': '9905',
'game': 'gru',
'user_group': '[1, 36, 529702]',
'user_condition': '[1, 36]',
'device_type': 'phone',
'device_model': 'TCL 5007Z',
'user_name': 'Minnie',
'fb_connect': True,
'event_payload': '{"competition_type":"normal","game_started_from":"result_flow_rematch","variant":"target"}',
'is_active_event': True
}
Please let me know if I m doing something wrong while parsing the data to JSON. Also, I am looking for examples to do data masking and run some SQL within Apache Beam4