Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
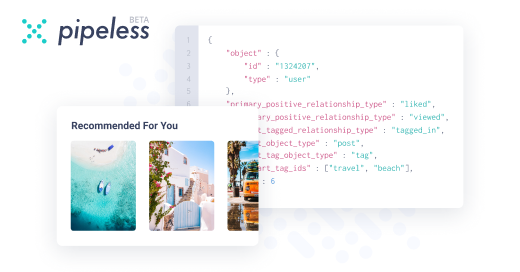
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "loops"
-
Colleague: "Python is slow..too much slow."
Then I look at the code:
Eight nested for loops, inside two of them, two function calls and three list comprehension. That function has 2 nested loops and two "objects.all()" Django method, plus a list comprehension too..
Yep, Python is slow.20 -
Revived my grandmas computer with Linux and she is happily using it without problems.
Today it was a good day.7 -
"How do I use a for loop?"
I mean I can't fault them for being open to being taught, but if you're working as a software engineer...and can't use loops...11 -
Please for the love of god name your variables in a sensible way! How the FUCK am I supposed to read your shitcode if you decide to write 6 (!!!) nested loops with variables each named by exactly one character. With no comments whatsoever!
I would rather crack password hashes than this nonsense.13 -
GUYS WE HAVE BEEN WRITING FOR LOOPS IN JAVA WRONG THE WHOLE TIME. Here is how it should be done correctly...
int i = 0;
for(;(i<10) ? true : false;)
{
i++;
System.out.println(i);
}
Jokes aside though does anyone have any more horrid ways to write this?20 -
Going to forums asking for help as to why my nested for loop wasn't working correctly after the first loop only to realise I was using the variable i for both loops...
Felt like an absolute twat4 -
Got the laptop from the job.
Sadly we will develop in .net and angular.
Machine has windows and i can't use them but whatever it's a job so i have to adapt.
Turned up the machine, needed 30mins to set it up.
Meh. I miss my vim and i3wm.
HELP6 -
Dear Whatsapp.
FUCK YOU
When i sit on my computer and use the web application, it means I WANT TO USE WHATSAPP ON MY FUCKING PC. If i wanted to use my mobile, i would DO IT. BUT I DON'T, SO I USE MY FUCKING PC. So don't fuckin tell me to connect my telephone to the internet so i can use your fuckin web application. I ALREADY GOT A HEADACHE FROM THE DOUBLED NOTIFICATIONS.9 -
GOD FUCKIN DAMMIT
I WILL FUCKIN KICK YOU ON YOUR FUCKING THROAT.
Programming Languages and Linux groups in facebook are a fuckin pain to watch.
Some people make groups so all can benefit and help each other, talk about mutual interests, BUT NO SOME FUCKERS WILL SPAM SHIT AND MAKE YOU WANNA SMACK THEIR FUCKIN HEAD.
THERE IS A FUCKIN FAQ SECTION THAT ANSWERS ALL THE FUCKIN NEWBIE QUESTIONS. WHY THE FUCKIN HELL YOU SPAM IF YOU HAVE NO FUCKIN CLUE WHAT THE HELL YOU ARE DOING?
You come to a python group and ask if it's possible to get context from a site. I'M NOT MENTIONING THE FUCKIN FACT THAT THIS IS A SIMPLY FUCKIN QUERY TO A SEARCH ENGINE ALSO IT'S MENTIONED IN THE FUCKIN FAQ. Let's move on. We tell you yes, there is BeautifulSoup for that. After 5 fuckin mins YOU COME AND MAKE A NEW POST THAT SHOWS YOU CANT FUCKIN ITERATE A GODDAMN FUCKIN LIST. I'm not pro either, i don't forbid you to learn, BUT FUCKIN LEARN THE BASICS THAT ARE PROVIDED TO YOU FROM GREAT FUCKIN RESOURCES BEFORE TRYING TO ATTEMPT SOMETHING MORE COMPLICATED. AND IF YOU NEED HELP PROVIDE CODE THAT WE CAN USE. NOT A FUCKIN PHOTOGRAPH FROM YOUR MOBILE
Let's go on the Linux groups.
SINCE YOU FUCKIN JOIN A LINUX GROUP YOU ARE SUPPOSED TO KNOW WHAT THE FUCK IS LINUX. IT'S A FUCKIN OPERATING SYSTEM RIGHT?
Then you spam shit like, UBUNTU OR MINT 5 MINUTES AFTER SOMEONE ELSE MADE THE SAME VERY QUESTION 30 MINS AGO. WHICH WAS ANSWERED AGAIN YESTERDAY.
"What are the benefits of Linux". NONE YOU TWAT, IF YOU NEED ME TO TELL YOU THE BENEFITS OF THE SYSTEM THAT YOU USE THEN WHY THE HELL YOU BOTHER.
Next.
You say you have problems setting up XAMPP. We tell you that since you are on linux better use LAMP. You ignore us and spam your fuckin problem with XAMPP. IM GONNA FIND YOU AND IM GONNA MAKE YOU CHEW MY FUCKIN SHOES YOU PIECE OF SHIT.
I'm not even mentioning the kali wannabe hackers.
Conclusion:
DO A FUCKIN SMALL RESEARCH BEFORE SPAMMING THE SHIT OUT OF STUPID FUCKIN QUESTIONS. AND IF YOU CANT EVEN SEARCH, LEARN TO ASK IN ENGLISH THAT IS FUCKIN UNDERSTANDABLE SO SOMEONE CAN GUIDE YOU ABOUT WHAT YOU SHOULD SEARCH
OH FUCKIN GAWD IM GONNA THROW MY LAPTOP OUT OF THE WINDOW8 -
I'm done with this fuckin shit.
Why it doesnt even work.
Fuck it. I will look at it tomorrow.
*after 5 mins*
opens terminal, editor:
- o boi, let's go again2 -
FUCK WEB DEVELOPMENT.
Seriously, what the hell. Things evolve so goddamn fast and someone new to the field can’t even grab something to start with. Once you start understanding something it fuckin changes and something else takes it’s place.
Fuck this shit, I’m out.18 -
So today I wrote CSS using for loops and if statements in scss.
You could say I was programming in style... -
I hate React.js with a fucking passion. It sounds great on paper, but once your project gets any size. The mental recursive loops of passing data around is insane. Not to mention keeping consistency a cross metric shit ton of components. How do you manage it?26
-
Fuck i love regex... managed to replace a series of loops and if statements with regex and cut execution time from 60ms down to 5ms13
-
I am going to create a new language called Yoda that is identical to Java but it has no exception handling and no for loops.6
-
Loop counter conditions.
10 loops for example in this scenario:
i<=9
OR
i<10
Was arguing with a co-worker all week over this 😂11 -
Keep your computers and laptops clean you disgusting fucks.
Went to my boss’s office to leave a document and when i saw his laptop i almost vomited.6 -
This is one from i was an intern
Me: writes some logic, using
for(String s : something)
Senior: holds a 30 minute talk on why short loops are bad and we should always use the traditional one
Me: ok retard
Me: havent used traditional loops ever since then8 -
Oh dear, a scaling problem I solved was replacing some Regex matching with simpler string functions. While I'm a huge fan of Regex, it's unreal how much performance they can suck out of some high-n loops...
I got about 120x out of some critical code thus making a CPU upgrade unnecessary.8 -
Just reviewed collegues code: 80 lines of if statements that are so long that I have to scroll sideways on my 27" screen. Just wrote the exact same thing in 5 minutes and 10 lines! If you don't know how to use loops in your code please learn something different and stop pissing off skilled devs 😡5
-
Dear Teacher.
Thank you for forcing me to use a windows computer for your lecture, even though i have told you that i don't have any windows machine.
Thank you for forcing me use 3DS max for your simple fuckin trash that needs 20 minutes of work, whilst i could have used gladly Blender.
Thank you for making me deal with that shit that i won't touch again in my life again just because you teach a simple lecture that isn't cleary for computer science which i and many people study, but for people who deal with graphics.
What more joyful i would have done rather than downloading 8GB of software to use once in my life
Thank you, you piece of shit.13 -
FUCK
Have you ever worked with people that constantly asks you what to do? People who are in the same spot as you, I mean.
- Hey, you should start debugging this while I finish this menu
+ So what's failing?
- I don't know man, but there's a bug
+ But where?
- You should look for it, I'm trying to link this to the controller
+ How can you not know?
- Do you know?
+ Where should I look for it? Here?
- ...
(One crappy solution after) + Here it is, I'm moving to something else
- ...11 -
The other day, I customer of ours asked me to try to improve the performance of an application of his in a particular method. The method in question taking more than 5 minutes.
I took a look at what it did in the profiler, and it shocked me. More than 100k selects to the database, to retrieve 116 records...
I took a look at the code... Scores of selects in nested loops inside other nested loops inside of... That seemed normal to them...
At the end after we improved it's performance it took 3 seconds...
What shocks me the most is that the customer is a developer himself, really knowledgeable and has an order of magnitude more experience than I do. Am I too anti "worthless database round-trips"? Is that normal? :S1 -
Im learning python(it's my first real programming language) and I finally understood for loops.... yeeeey6
-
The time I learned loops and I was fascinated by the fact that I could do 100 operations in a few lines. I was hooked.7
-
Started a new job this week.
Just learned that my manager strictly prohibits while loops and "if else" statements.23 -
Been lurking for a while, figured I would give a rant a go now that I have graduated college!
So here we go, this was the only technical question asked on my second co-op interview. Ended up getting it.
Interviewer: "Do you know any loops?"
Me: "Yes....?"
Interviewer: "Which ones, can you name them?"3 -
Damn, my boss added me to a "almost" complete protect with a bunch of spaghetti code in a language that I don't know more than the syntax of for loops and declaration of variables (swift) ..... I'm really fucked6
-
When you look back at Code from your 2nd year of university...
Using while loops as if statements to check for vowels... *shudders in fear*5 -
Who the fuck writes a 200 line method with 52 if/else statements, 3 try-catches, 6 loops and only 1 comment saying //Array of system records. No dipshit I thought that was a Fucking interface. What happened to the whole keep it simple notion?!5
-
Developer 1: Stop changing all my loops into switches!
Developer 2: if you'd learn when to use a switch I wouldn't have to change it for you!
-My 2 devs argument today
#officechat #good2betheboss8 -
Everyday I change all of my colleague's i++ in ++i in for loops, and he changes my ++i in i++. This goes on forever...11
-
When I just started my software engineering course in college, we had a group project every semester where we would use the skills learned during that semester to make a certain product or program.
For the semester in this story, we were tasked with making a reservation system for a campsite. Visitors would be able to select a free spot, and reserve it.
The spot reservation screen would be a map of the campsite, and visitors would click on the desired site on the map to select it. Sites were neatly laid out in a perfect grid.
My task in the group for this project was my favourite position: yelling at people for poor code quality. And boy did I get to yell.
Any semi competent programmer would probably come up with two simple loops to generate all the buttons (something like 144 buttons), one loop to fill a row, and then another to go down the rows until all were filled. Some other similar functionality in the program was solved this way.
However, my classmate that was responsible for this part of the code wasn't a big fan of concise programming. So instead, he wrote 144 functions aptly called `generateFirstButton()` all the way through `generateHundredFourtyFourthButton()`.
*what*
I called him out on his horribly smelly code, and his retort was "But it works, and now you don't have to think about complicated loop logic".
I rewrote the class and reduced it from ~1150 lines to about 20 lines.
He didn't pass the exam.2 -
We used to write infinite loops with "start cmd.exe" instructions in windows batch, converted it to a executable, gave it an epic vector icon and tried to sell it to 5th graders on CD as "an epic virus that will make your teachers go insane".
And some of it actually got sold.8 -
I used to prefer, think, and code in loops rather recursion.
Now though somehow it's the other way around...
And I have to assure myself why a piece of code I wrote won't cause a Stack overflow... and that if I really had to I could convert it back to a loop.
Haven't done it though for a awhile tho...7 -
Watching Limitless on Netflix and I just love the "hacker" code animation loops with multiple terminals open going on in the background xD5
-
Python does not have ++
Feels so different as I used c c++ and Java!
Just can't write while loops with x++
😭4 -
Boss interviews an hot girl
Me:"well how was she ?"
Boss: " she doesn't even know loops"
Me: " I'll help her ! We can create many loops together " -
Because fuck logic.
'This returns a lot of rows
myAdapter.SelectCommand.CommandText = "SELECT FROM tblNews order by DateAdd desc "
myAdapter.Fill(rs)
'He only wants the 4 latest articles
For index = 0 to 4
'Do something with the data
Next
How some people manage to still have a job is truly fucking amazing.4 -
I remember the days when your only problem is creating an asterisk pyramid with for loops. Unlike now when you are thinking how idiotic a user can get.
Good old days1 -
!rant
So family doesn't really know coding. Just me and my little sister. But then my dad suddenly made jokes with loops and stuff. I have no idea where he learned it. They're not actually accurate but I really love it when he does that.2 -
My IT teacher in high school. Got me into programming, helped me take programming from a hobby to a career and is still helping me today. Honestly one of the coolest people I know.1
-
Earth keeps rotating
Seasons repeats
Life goes and come back
Machines in factories doing repetitive job
You sleep, you wakeup, you eat daily
... and y'all think loops are bad?2 -
Things to do while you encounter a bug and haven't resolved in some hours:
- Leave it and get clean air so your brain relaxes.
What i do:
- Stay there trying to figure out why the fuck this shit doesn't work and considering falling from the window a very good option1 -
Spending a few hours messing around with regex and for loops to parse HTML just to SO and learn about BeautifulSoup. Why was my life wasted so!2
-
My loop checked a variable, then the next 5 nested loops checked it again, then started over. It was a hard coded variable I forgot about.
I just made my code OCD -
*Program crashes*
Colleague looks over my shoulder : While loop?
Me : ...while loop.
It's been two years...my brain just refuses to write non-infinite while loops. I tend to avoid them as much as I can cause every time, without fail, first time I create one I crash something.2 -
> you are a teacher at a university
> you are supposed to teach advanced things to students
> your slides are screenshots of the book that you told us to get
> you sit at the desk and just read the slides
> you don't even try to win student's attention
> students prefer not to come to your lectures
> you wonder why a lot of people fail your subject
> WADAFAK
> ??????????5 -
Somebody pays for this domain 👍
Translation: www.if-loop.de
There are no if-loops, just if-statements! -
When I was really new to JavaScript, I wanted to create an image gallery with images which I stored in a MySQL database. Well, I did not really had a clue how to load all the image sources into my JavaScript to load the images. I also didn't know much about fetching an endpoint of my website to get the data asynchronously.
I also wasn't a good database architect at that time and my database had an image table wich was for the gallery. Within this table there were multiple columns for one image slider (there should be multiple sliders on one page in the gallery (I know... 🤢)).
So I ended up writing an PHP loop wich printed Javascript loops for each row in my images table. Within my JavaScript loop I created the sliders and set the images.
In my defense I can say: It worked. 😅
It hurts to remember this. And I hope you won't judge me.2 -
It was my thesis defense and I've made a pretty complex algorithm of sorting out data to their respective tables. One panelist told me that it is not an algorithm but just a collection of for loops and if statements. An algorithm should contain mathematical equations he said. I facepalmed during the course of interrogation.
-
Historically I've done Java but have recently moved to C# and got the book to do a cert.
Who thought it would be a good idea to start chapter 1 with threads, concurrency, asynchronous calls and PLINQ, then covering boolean expressions, if statements and loops in the section after??6 -
FUCK WINDOWS FUCK NODEJS FUCK ANGULAR FUCK VISUAL STUDIO FUCK POWERSHELL FUCK ALL THAT FUCKIN CRAP WHY THE FUCKIN HELL IT SHOULD BE THAT HARD TO INSTALL SOME SHIT AND START WORKING LIKE A NORMAL PERSON WOULD DO JESUS FUCKIN CHRIST I FUCKIN HATE THAT OS FUCK THIS SHIT10
-
When you spend half an hour long at your code wondering why your do while loop only works once.
Then you realise there is a return just before the end of the loop.
Today was not a good day2 -
I always thought the hate on senior developers doing stupid stuff was exaggerated... Mine just pulled an entire table, then used 4 for loops to reduce the records by criteria... I don't think he knows what the where statement is in SQL!1
-
In an encryption-module, I had a bug, that caused my PC to crash, every time I tried to encrypt something.
Turns out, the loop, that appends
0-Bytes to the string, to make it Block-Cipher compatible,
Had an logical-bug in its exit-condition, that caused it to run infinitely and allocate an infinite amount of memory. -
So a guy trying to convince me that vim is bad, just told me that jetbrains is better than vim, which he accused as dead software, because the jetbrains website is better than vim website.5
-
uni prof: "you can't make an infinite for loop. Infinite loopsnare only possible with while loops"....
I think it's not what he meant, but it was a while ago and I forgot the context, but multiple students made a 'what the fuck?' noise.5 -
Been wondering lately. Do Python devs use type hinting? Shall i start implementing it?
I find it this way, a bit more clear about what the method is doing9 -
Why people are saying Python is an easy language? I mean yes, if you write c/java code with python it may seem easy, but writing proper python code, in a pythonic way is not so easy.
SO DON'T TELL PEOPLE THAT'S IT EASY LANGUAGE because new commers later they come with absurd code and ask people to fix it.7 -
Came to my parents house for the vacation period and forgot to push the code i was working on my computer. Now i sit with my laptop crying.4
-
Fuck NodeJS. I don't want you to be asynchronous sometimes. My gahwd I need like 3 deep nested loops just to get you to make a request and compile a damn JSON of requests.
I'm learning Golang goodbye forever node.15 -
Actual production code:
while(1):
//A few lines of code here with a conditional break.
while(1):
//More code.
Have you ever just had nested infinite loops...4 -
Please, stop using `i`, `j` and `k` in loops... specially if they are nested, help the fellow programmer after you29
-
Me and one teacher i got, both Linux enthusiasts, decided that we should create an open source community and spread the word to other students, teachers and stuff from the university about Linux and open source in general.
First meeting went well, we got quite an attendance, people seemed curious and willing to learn.
Second meeting is tomorrow, and we decided to show them some Linux distributions and DE.
Guess what, we can’t decide what to show them.
He is a pure Debian lover, i use both Debian and Arch.
He insisted on Mint since he didn’t want to scare them with Debian.
I said that we could show them Manjaro.
So what do we do?7 -
New programming research project from Microsoft called Bosque.
A programming language without loops.
https://github.com/Microsoft/...9 -
Browsing Reddit for weird porn back stories and you stumble upon the efficiency regarding loops
P.S. - the comments has more9 -
Anyone else just nest a bunch of ifs and loops and feel absolutely disgusted but it works so you don't want to refactor it?5
-
I have a course at my university about personal data and it feels more than a law course than a computer science one. I asked the teacher why do we have to be taught that subject and how would we be able to use what we learn in real life and she got triggered, telling me that with just by coding I won’t achieve anything and i have to learn more topics and if I didn’t want to be taught this subject why did i choose the university.
I just made a question you fuckin butthurt, chill the fuck out.3 -
While calling a tech company:
10 *music starts playing*
20 "You're second in queue"
30 GOTO 10
Is it so fucking difficult to let the music continue so I won't have to hear the same 10 seconds of the beginning of this already annoying song?!
When I finally get connected they tell me my order won't be in stock for another month... I just want my beautiful monitor 😭1 -
// O(n²) complexity
for(x;y;z){
for(a;b;c){
}
}
Dev's argument: "We use this everywhere, as long as it gets the job done! Time is money!" How ironic..
So you would rather make your processing speed suffer for the sake of saving time? No, clean code doesn't matter. No, we should not waste time spending even a mere microsecond thinking about writing better code or at least consider it. No, we should just vomit out bad code at top speed. Good idea, guys. Idiots everywhere..6 -
Thought about playing a bit today.
Booted windows. Got 2 bsod in 5 mins.
Wiped out windows from hdd.
This was the last step of my "gaming" era.2 -
Dear Facebook.
FUCK YOU YOU FUCKING CUNTS.
The next time you upgrade your piece of shit application on iOS and remove stuff from their place which makes me search all the way your crap battery vacuum application until i fuckin find it i will come to your office find that dumb zuckerberk and push the fuckin chair he sits at down to his throat.
Same goes for your retarded website.
Suckers.1 -
One of the worst loops of my life
(can't find glasses cause I can't see)
(can't see cause I don't have my glasses on)1 -
I’m 20, but i use vim, not because i feel superior or more clever than others. At first i hated it but by the time i said im gonna learn using that sucker which i did. I’m writing Python and thought maybe by using vim i add nonsense complex to my life. Tried atom which i was using before. After 10 minutes i gave up and opened vim.3
-
Had to fix all bugs of my colleague this night because our client was not happy.
Before he joined us he worked as a database admin and now he wants to learn web dev and coding. But he did so bad mistakes like endless loops or requesting api 5 times.
In so tired now, happy when its christmas -
My whole workflow for HTML is frustrating. I rather write pug, because it has mixins, includes and isn't that fucking repetitive. But to write pug within Laravel blade views, I have to preprocess it too blade. Then sometimes the syntax gets crazy because of escaping blade loops like an insane guy ('| @foreach($foo as $bar)'), but you get used to it after time.3
-
WHY THE FUCK THE NEED TO USE Visual Studio.
Well, in my university, for some fuckin dumb reason we are taught to develop a simple fuckin web form in asp.net.
Thing is, VS is so fuckin powerful that it's a huge overkill for such a simple thing. What is even worse is that, WE DONT FUCKIN EVEN CODE IN C# we just drag FUCKIN COMPONENTS HERE AND THERE without learning a single thing
But okay let's move on. I'm a linux guy, which mean, I CANT FUCKIN USE VS AND CANT EVEN PRACTICE THOSE DUMB SHITS and that means i won't fuckin remember a single thing.
FUCK THIS SHIT2 -
Should I be ashamed?
I hardly ever use "i" as a counter in for loops
I most likely use "k" and "j"; "i" stands for "index"23 -
Finished exams, in 3 days i have a flight to my parents house to see them.
Before that, thought about comming to my grandma's home, which has a beach nearby so i can spend 1-2 days relaxing.
Came here. No one is home. I don't have keys either. I'm hungry too.
Fortunately the wifi signal is ok.
Sat in front of the door, and opened my laptop.
Time to start migrating my projects from duck to static typing and practising my ability to write python code with type annotations until someone comes home.3 -
According to people here, ternary operators. I don't care what I'm doing, if I can use a ternary operator instead of an if, get ready for it!
I guess implicit brackets on conditionals and for loops if it's a single line. Anything that saves me typing boilerplate, but it's still readable, I'm gonna do it.1 -
Just learned how to write a for loop in kotlin and I now want to burn Java to the ground. When I first learned for loops in Java my brain almost imploded.2
-
There's always been the debate about omitting braces for single line if's and loops, but today I learned the C compiler actually allows you to do this:
for (i = 0; i < x; i++)
for (j = 0; j < y; j++) {
do_something(i, j);
something_else();
}32 -
Public transport is slowly getting quieter and quieter. One side of my enjoys the silence and space, but the other side wishes I had the option to not take it either.
Everyone WFH, I envy you...2 -
So I made my first rust program with just a quick look at the docs on for loops. 20 minutes from nothing to exe. Just fizz buzz but still. Took me 4 hours to get C++ compiling.3
-
Configuring apache is so fucking repetitive and inefficient. No for loops. No arrays. Just repeating damn near the same lines over and over and over again.
Oh you want to listen to 20 ports? I hope you like copying and pasting.4 -
So I broke my beloved Fedora Dist. instead of doing a little "sudo chmod -R 754 ./" on a development folder, I did it on root folder ( / )
Now, OS crashed, and I'm getting infinite loops when booting.
But I'm not even mad. I'm impressed :o7 -
When you're doing a math problem at school and you can't solve problems with loops and if statements2
-
I once saw a DBA using two for loops in PL/SQL to join two tables. I wonder if he knew about the "alternative" way to do that...2
-
Started out with python, while meaning to learn javascript.
I am now competent in python. Im still not sure how it happened.
Started python because I got tired with doing repetative calculations by hand. I think I had like a phobia of problem solving with nested loops. any time I thought a problem would require nesting, especially more than one nested loop, I would just avoid doing it, or end up doing it by hand.
Wrote so many goddamn loops though in the process of exploring graphs, doing things by hand seems like a nuisance. Thinking in loops has its own zen or something.
Now I just need to get over my fear of json-based CLI-enabled configuration-over-convention.1 -
Why some single letter variable names looks ugly to me, for example: c, i and j (and even k) are the most used (mostly in for loops) but does not look ugly.. why?
Ugly
for (int a = 0; a < 10; ++a)
Not ugly
for (int i = 0; i < 10; ++i)24 -
during a programming introduction course on loops my pals started writing `for` loops instead of `while` loops like so:
`for (;expression;) {}`
spent weeks explaining why it's wrong. needless to say, they still do it. had to hinder myself from ripping my hair off my head11 -
vscode for now. I swap editors when I get bored of one. All editors have something cool to them. Except Vim. Screw Vim.1
-
Colleague (Lead Engineer): Hey, check my code. I'm trying to group a list of Request objects by their id. Something is not working here
Me: * saw his code, had a lot of shitty loops, called all for a quick meet, changed his shitty mess to one liner
list.stream()
.collect(Collectors.groupingBy(Request::getId))
Walked out like a boss*
😎 -
I remember the day when I was in first year college. We were tasked to make a c++ program that will print a diamond pattern. Didn't know that you could achieve that with for loops.
What I did?
A hard coded one.
What I got?
An F -
Turns out my colleagues don't understand how useMemo works. They would try to memoize a value and then, in the dependency array, use an object that gets rebuilt on every rerender.
Good job, guys. I wonder how many silent infinite loops we have because of that shit.1 -
Coming to the end of beginners python and added a new loop to a number guessing game and now my elif is invalid syntax.19
-
So I'm writing a function in Unity3D that walks a rectangular grid. At one place in the code, I got the x+y coordinates backwards, which caused the function to infinitely loop between two coordinates.
Not seeing a way to kill the loop, I looked it up on Google. The suggestions I get are. . .
1. You need to kill the Unity3D task and lose your edits because the environment and the player run on the same thread.
2. You can pay ten bucks for an extension that lets you break out of infinite loops.
3. You should really avoid writing infinite loops. That's just bad form.
SERIOUSLY?1 -
Fucking hashtables...I forgot that removals can screw with the probing sequence, causing later lookups to "randomly" fail after hundreds of operations and elements.
Spent 4 hours staring at 3 while loops and data sets of hundreds of key value pairs trying to figure out why one giant data set worked fine but the other failed on some lookups.2 -
Yo vim what the fuckin fuck.
I like vim, i try to use it as much as possible since i feel more confident with just using a keyboard BUT WHAT THE FUCK.
I am developing an application to improve my python skills and I chose vim to do so. I made some “big” changes today to it using vim. Every time i made a change that i had to test, i was saving it with :w and then running it on my second screen. All good until now.
Then i wanted to make a minor change using vscode because i thought it will be easier there. Anyway, i used :x, opened vscode AND MY CHANGES WERE REVERTED to the first condition my file was when I opened it today.
Vim is awesome, maybe it was all my bad, but how the hell did that even happen?2 -
i'm afraid that having discovered the power of multithreading has made my code worse.
case in point: me has to calculate an unknown 3rd point of an equilateral triangle many, many times. however, me doesn't get the formula, so me goes ahead and loops over all possible coordinates until it finds the correct one.
yep, it's definitely gotten worse.2 -
Can someone come and clean my desk? I not on the mood and my code to clear the desk seems to be stuck in a wtf loops.4
-
To the people that mistake i and j in nested loops: Have you considered giving these different names?18
-
Made a small text adventure type game with C++ when I was a kid. Was great to just play that over and over and revel in my mastery of if statements and while loops.
-
i had to write some js and css / html for a small project that i work on Django.
GODDAMIT I FUCKIN HATE IT MY HEAD HURTS WHAT THE FUCK IS THIS SHIT
FUCK IT
LEAVE ME ALONE WITH MY GOD LOVELY PYTHON
OR ANY BACKEND LAGNUAGE(except php of course)
jesus christ i almost threw the goddamn pc out of the window.
fuck front end.11 -
Too many people seem to think that "goto" in C is the worst thing you could do. It's an abomination, never use it! (Not something I've seen here per se, just generally around)
Challenge:
Break out of a loop inside a loop without using goto, and make it look pretty. Seriously, I don't think it can be done, and goto just makes it so clean!7 -
Fuck you MATLAB and your shitty inefficient for loops. Now I have to rewrite most of my code to use matrices instead of structures cause you take so long. Fuck you and your stupid ability to scale my neural network.....who needed sleep anyway6
-
How do I become good with functional features(map,reduce,filter,zip,flatmap) in Javascript and Python?
It feels so alien. I'm so used to writing plain old loops.
Reading and undersranding this kind of style in other people's code is really hard for me, especially if all this is happeining on the same line.4 -
While while is still useful sometimes, all hope is lost for for.
For each for each that I use, I love it more and more.15 -
CNC software
Hey guys
what do you guys use to control your DIY CNCs?
I'm using UGS, Universal Gcode Platform, but it has a slight problem... doesn't work with functions.
Meaning, I just had to write a 75 lines program that could be an 8 line program If I could use loops and GOTO...
Also, what CAM software do you use?13 -
that moment when a fellow dev asks you a web dev ( PHP ) for help and you see
... -03 -0fast & funroll fruity loops ...
im not surprised that Gentoo box died ... -
- Hey, could you help me understanding your method? I'm trying here to implement it on my side but it doesn't work
- I'm not at home right now and don't remember the code i wrote. I will look at it when i get back home
- Ye but can you explain it briefly?
- I JUST FUCKIN TOLD YOU I DONT REMEMBER IT EXACTLY, I AM NOT AT HOME AND I DON'T FUCKIN HAVE THE COMPUTER WITH ME. WHAT HE FUCK WAS SO DIFFICULT TO UNDERSTAND?2 -
Pretty tired, plus working on something that at some point needs 4 or 5 for loops inside each other
End result: for (i=0; k<=Nspecies; ++j)
Mhkay time for coffe -
Why is vectorization library faster than hand-written for loops ? I mean, somewhere down the line, the matrices/vectors must be multiplied (or any other operation) and thus be one-by-one (for loop??) calculated and stored.
Why is it then faster to use these libraries than just manually writing for loops all over the place ?
I guess some low level magic (OpenBLAS ?) goes on there but I just don't see it..
P.S. [Would have posted it on stack overflow but I'd be ripped apart so I'm pioneering new ways]8 -
Worst mistake? Well nothing to bad since it only affected me. Tried out of curiosity to instantate an object every frame in unity that ran an infinite loop every frame. It crashed not only unity but also my computer resulting in about an hour of lost work :P
-
Sins? All of em'.
Infinite loops of recursive callback carnage, just because I like to watch it burn. -
Recalling the the time I was discussing a web dev based assignment with a classmate( And I wouldn't even go as far as to call it web dev, it was just making connect 4 using js and node).
Me: so did you get to implementing your game with firebase.
Classmate: no, but hey did you use loops for making the board?
Me: ?? There were like 50 elements ofc what did you do.
( Sigh* to think it's going to this)
Classmate: copy pasted
Yup 50 elements copy and paste. like the person I was talking to wasn't even incompetent I mean he had like a 3.5 gpa, how is there any correlation between this stupid number and how they actually code if you can't even code loops. The dude was a 2nd year student. And this wasn't even the only person I heard this from.
Apparently the TAs had to post about how to use loops in Js before we had our lab exam cause so many people copy pasted instead of loops, to think that would ever have to be the case.
The future is not bright. -
Logging into my schools blackboard using selenium is only redirected on school WiFi
Outside school WiFi I go directly to the site. But on the school WiFi it pauses on a redirect page with a link that loops back to the redirect when grabbed by selenium And selenium fails
Fucking hell2 -
ASUS thanks for putting the power off button on the top side of the backspace. I mean what could go possibly wrong you fuckin fucks!
-
Had to do the FizzBuzz test in PHP. Proceeded to creat a range(1, 100) before the for loop instead of using the loops own index #. Worst part is I realized what I had done in the parking lot after I left. They asked me multiple times how I could optimize the code too lol.
-
Anyone know of OSSU? It seems cool, but I don't know anyone that has any experience with it. Would be doing it part time to fill all the gaps of my self learning journey5
-
Sitting listening to music, thinking about some feautures that i could code for practising my code skills.
Phone rings, a girl that i know asks me if she could say at my place.
Can't deny, i said whenever she needs help i can provide it.
My git pushes are delayed for tomorrow.
Sorry for betraying you Vim.1 -
Things forced upon me throughout my life that I hate with a passion.
Football (I mean soccer)
The (fucking) Beatles
Religion
Microsoft
JavaScript
Most Clients
Things I can't get enough of (in no particular order)
Asian Food
Dirty Loops
Sleep
Playing Bass
Travel
Time1 -
Me: How to use those retarded promises in typescript
Every result on internet: 20 pages article. Let me introduce you to angular, first you install npm and node then you create a project, name it whatever you want, then we create a file.
Promises motherfucker, how those work? Can you simply write about that?1 -
Just wasted 3 hours because i was manipulating the context dictionary in django of a different view that i was actually checking.
When you see there is no answer to whatever you search, move back and go for a walk.
This shit drove me nuts.
Now i need my brain to calm down.
Still wondering why my mom thinks i'm a clever guy. -
Just went over some of my old horrible code from before i started studying. I litterally went from 104 lines of if/else statements to about 15 lines with loops. Then down to 2 lines with lambdas...
I saved 102 lines of actual code. And the runtime! I had a loop count to 10000 with no body! It helps to study apparently :/ -
Don’t fall for the “language A is better than language B” -it’s all just syntax (within the same paradigm). Learn higher concepts such as: data structures, abstraction, loops, variables, conditionals, functions.
Only then can you decide that your favourite language is better than anyone else’s...5 -
First development job I had, I wasn't allowed to use LINQ. According to my boss, it was the "lazy" way. So many for loops :(1
-
Trees -> declarative programming
Loops -> functional programming
Sequences -> imperative programming
Graphs -> dynamic programming
Good mapping, yeah or no?15 -
Sir i am a newbie student to CP. I have recently started CP and just know array , strings , loops , function and basic libraries along with logic.
Where and what roadmap shall i follow so that i can get rated nicely and quickly on codeforces?
(Currently about 700 rating).7 -
I know that when a deadline is present, things like cyclomatic complexity might be something that is a left aside. But I hate so much having to troubleshoot a method with 15 levels deep of nested if, try-catch, loops... Fuck!3
-
Okay so update/JS pt2
This is just me throwing my thoughts down and some questions
I've been practicing arrays/objects and loops more and I'm getting more understanding it helps that you can do them both at the same time. But like I need more looping techniques (if that makes sense) like instead of always using for(let i = 0; i <= x.length; i++) and I havent completely learned how or when I use for(x in y)
Questions.
• what's the difference between class objects and objects that look like a python dictionary
• when should I use classes over the other kind of classes
• any good resources and projects I can practice with loops cause I'm kind of running dry on ideas
and I dont wanna google cause I barely already have no social interaction2 -
A database fetch. All rows at once. Not that many rows, maybe 50.
But oh boy when someone forgot that the repository is wired to magically inject SQL that joins other tables and does ineffective loops to create thousands of objects in the background.
Been fun finding memory hogs in the codebase. -
Last week I had a meeting to get us all on the same page for today's prep meeting... Tomorrow we have the actual meeting to be followed two hours later by the meeting review meeting... Culminating in decisions that drive the direction of a set of upcoming meetings... Which will all have prep meetings and some have further review meetings...
I'm so excited...2 -
I started my coding journey with JAVA ! I l grasped the basic concepts like LOOPS TYPECASTING ARRAYS etc. pretty well but failed to cope up with stacks , queues . So I switched to python and completed the Python Bootcamp from Udemy and now I am pretty confident in python . So should I try to learn Java again ?2
-
While learning Java back in campus and you were the only one who could nest some for loops and create some pyramid stars and the lecturer was on you to(Java Ninja) explain it to fellow classmates that felt good.
-
Using API to get todays weather.
The value i get is 293.some other decimals.
Well, i guess i have to manipulate that float.
Converting it to string, moving the dot and convert it again to float so i can have the number in a form i need it, which is 29.decimals.
Hm...
Re reading the docs, and i find out the value is in Kelvin.
FUCKIN KELVIN
WHO THE FUCK USES KELVIN3 -
First assignment after I learned about if-else-then and for loops: draw swastika with modifiable width variable2
-
Apple's thoghts: it's better to create shortcuts using the new ios12 app to turn on/off completely wifi/bluetooth rather than selecting it from control center like it was supposed to function
10000 IQ3 -
1 of the many windows rants i will make since i have to use that on work's laptop.
Let's start.
20GB for an IDE? what a joke.
Seriously MS. Are you fucking retarded? You have a fuckin retarded windows store, and i need to open it 3 times for it to be functional? Fuck you.
I want to change the default browser. YES I FUCKIN WANT TO CHANGE IT, STOP FUCKIN ASKING ME 500 TIMES IF I'M SURE ABOUT IT AND DO THE THING I TOLD YOU TO DO. Fuck you.
2GB on startup? Thanks, appreciated. Miss my 250MB on my linux laptop. FUCK YOU.
Did i fuckin tell you i want to sync the onedrive? I clicked that shit to disable it and you decided to sync the fuck out of it? Fuck you.
Candy crash and other retarded games? LOL, can't say anything. The laptop is from the job, i won't bother uninstalling that shit. In any case MS would reinstall them again. Fuck you.
Fans at 100% for no reason and battery draining like it's a cold drink that drinks someone who is thirsty? Thanks MS, very helpful. Fuck you.
Powershell? Well i leave that. Whenever i remember how slow it is i want to throw the laptop of the window. Fuck you.
Notifications? Thanks, it's very good thing to spam them for no fucking reason. Fuck you.
Skype? Who the fuck told you to install it? Fuck you.
Well i won't say anything about their fuckin updates. Every one knows how retarded and piece of shit they are.3 -
Exams are done, i passed some subjects that made me almost drop out.
Felt good. Now if i manage to do well again in exams i may finish the uni on time.
And now here it comes. One of my professors saw that i was coding my self in contrast of the 90% of other students, and with 2 more guys from my year, suggested us to his friend that owns a company, so we could work there.
I went there, talked about the team and the product we have to do and it seems that for now the only developers are me and 1 more girl and 1 more guy, all new commers, not even juniors.
Shiet. The team told us not to be worried since they will be our instructors and help us out and if we need more help they will hire a senior dev.
Not sure how i should react to that.
I do that mostly for experience so i can leave the country when im done with uni to go to estonia holland or finland.
One more thing, we still don't know what languages we will use and even though i told them that im pretty good with python they seem not to consider it at all. I'm the only one of the juniors that has actually made projects and coded on his own, not with university projects.
Also so that all other employees use windows machines.
Sad.
Hope all that goes well.1 -
Why I love to code?
1. The only thing I feel like I have control over (thanks to control loops XD).
2. Feeling like god when my code works.
3. I just love it, no reason needed, just pure love for it.1 -
Maybe it's just me, but:
1. Never works on first try:
doStuffWithWhile()
while (someShitIsNotOver)
doShit()
2. Works like a charm:
doStuffWithManualLoop()
doShit()
if (someShitIsNotOver)
doStuffWithManualLoop()6 -
What keyboards do you use?
Well atm i use a cheap mechanic keyboard which i got since my last one died. I can afford a new one and looking for options. I prefer tenkeyless keyboards. What do you use? Mechanic? Slim? Anyone uses mac keyboard with linux? Any suggestions would be very helpful.5 -
Just spent 30 minutes trying to work out how to do my dev terminals rendering in it's main for loop...
Only now just realised I should probably use 2 seperate for loops and rendering layers for the background and text... Well done dickhead -
Entropy wins. More useless accumulated historical nonsense in each programming language. Complex software stacks impossible to reach the bottom. However C and C++ still there possibly even some Cobol and Fortran zombies. And we devs still writing our ifs and loops for that kind of legacy stuff.
-
Does anyone here know how to set up windows 10 bootloader files?
So I wiped my laptop clean, installed windows, installed manjaro, and deleted window's ESP for shits and giggles. Then I tried using bcdboot to restore bootloader files in the ESP I put GRUB in, which got windows 10 on the GRUB but selecting it loops me back to it. Whatdoido.6 -
The fuckers that made my university’s website are absolutely fuckin trash. I’m trying to make a program that gets the page and creates lists with the names of professors and some more info and i fuckin can’t because they are retarded.
How bad can you be that you cant even fuckin create a simple html table with their fuckin names in it so when i scan it i fuckin get a name of a professor and an email of a different one attached to it. FUCK OFF2 -
The moment you decide to let YouTube just play what's recommended and after 4 videos it loops over the same 5 videos. Looks like I need to setup remote control on my phone now :( bed wasso warm..
-
I’m fucked off tonight.
I’m having to pull a very complex data set out of dB and loop through results in a table.
Easy, done, but one of the Columns I’m pulling out needs further broken down. It’s a comma delimited string.
I can’t get the data, and inside the same loop explode that string so that the contents can be handled independently.
Raging! I have foreach loops inside foreach loops and arrays inside objects that are inside arrays.
I’m going to bed furious.2 -
Could it be, that we use i for incremental loops because it's an abbreviation for iterator (or increment)? 🤔 Why haven't I though of this sooner?!8
-
It's so easy to use for-each loops instead of if-else chains, yet when I write a system in for the first time, I always find myself doing the latter.
"It's placeholder code" saves me every time as long as I rewrite it later.6 -
for some reason twitter showed as the first trend the word epiphany and the first thing i thought about was the web browser.
Was pretty good and light but missed a lot of things. -
Are there any certifications worth focusing on? I'm fresh out of high school, doing an internship at a web dev company.
Everyone I've asked has told me to focus more on experience and the things on my github to prove that I can code, but I'm pretty sure have some certifications will help me somewhere in life.
I've looked into Cisco, Microsoft, Google, AWS and many more, but I have no idea where to start.
Any help will be appreciated :)1 -
Trying to figure out what the API your company has been using does with 2 pages of documentation sucks! The explanation given to me was also pretty stupid: "So... you click here and then... uhm... this link, and uhm..."
-
void main()
{
if( isWeekday() ) {
if( alarmRinging() || kidsKnocking() ) {
startMorningRoutine();
escapeToOffice();
while ( !meetingsInProgress() ) {
code();
} //meetings interrupt the whole day, every time...
escapeMeetings(); //Go home.
startEveningRoutine();
} else {
sleep(1);
}
} else {
playWithKids();
doYardWork();
if( kidsSleeping() ) {
sleep(1);
}
}
} //end main. Like a microcontroller, this forever loops. :) -
Do you have multiple screens? If yes, in what orientation?
I'm personally using 2. 1 horizontal and 1 vertical.
The vertical one is nice for long documentation and the horizontal one is mainly used for gaming.12 -
Why are there so many template languages? Do people enjoy them? I certainly don't.
What is the problem with just using functions? E.g. using https://j2html.com/ or http://www.lihaoyi.com/scalatags/
You can do code reuse with functions, for-loops, classes, interfaces etc.
Why are they avoided in Spring, PlayFramework and similar? -
when manager recommends you use the wrong role for a job and then it loops all the way back they give you feedback when fixing it that they thought you were using the correct role1
-
I had spent all night writing a series of nested loops, my dream was me circling in an old above ground pool like I would as a child. We used to try to make a whirlpool by trying to run along the wall.
-
I am a student from IADT and I do not understand for loops and think that I failed my programming test7
-
What's it called when you count down to 0 for your versioning instead of up. Once you get to 0 your software updates freezes. I tried searching online but I keep getting counting for "for loops." This is eating at me lol.
-
I want to follow Ruby-related news/resources like Twitter, blogs, Medium etc so I can keep up with latest stuff or simply adding to my knowledge. The problem is I just don't have any idea who to follow and what good blogs or website are there. Anyone?1
-
Why does every programming language have to have so many different ways of doing the same thing? I mean, come on, do we really need both for and foreach loops? And why do we have to choose between switch and if-else? Can't we all just get along and use the same damn structure? #FirstWorldProblems30
-
What do you guys think about dopamine driven feedback loops of social media. Getting likes comments on the post gives you dopamine hit making you contribute more content!?!!! People are now willing to pay(to do anything). Beast is getting feeded!?!!1
-
- "what does that function do?"
- "I don't know"
- "how you don't know !? you are maintaining the project."
- "how do I know what 'ConvertFromDataTableToOther' will do !! it has two inner loops that exchange some columns!"
When CEO is doing code review ..